diff --git a/.env b/.env
index a01d747..622333d 100644
--- a/.env
+++ b/.env
@@ -4,7 +4,7 @@ EXPOSED_PORT=1026
# Orion LD variables
ORION_LD_PORT=1026
-ORION_LD_VERSION=1.5.1
+ORION_LD_VERSION=1.6.0-pre-1572
# Scorpio variables
SCORPIO_PORT=9090
diff --git a/FIWARE Working with Linked Data.postman_collection.json b/FIWARE Working with Linked Data.postman_collection.json
index 8af82a3..8b1dee7 100644
--- a/FIWARE Working with Linked Data.postman_collection.json
+++ b/FIWARE Working with Linked Data.postman_collection.json
@@ -1,9 +1,11 @@
{
"info": {
"_postman_id": "0bffb109-7af9-4ce3-b269-a53cb1e5c8e8",
- "name": "FIWARE Working with Linked Data",
- "description": "[](https://github.com/FIWARE/catalogue/blob/master/core/README.md)\n[](https://www.etsi.org/deliver/etsi_gs/CIM/001_099/009/01.03.01_60/gs_cim009v010301p.pdf)\n\nThis tutorial teaches FIWARE users how to architect and design a system based on **linked data** and to alter linked\ndata context programmatically. The tutorial extends the knowledge gained from the equivalent\n[NGSI-v2 tutorial](https://github.com/FIWARE/tutorials.Accessing-Context/) and enables a user understand how to write\ncode in an [NGSI-LD](https://www.etsi.org/deliver/etsi_gs/CIM/001_099/009/01.03.01_60/gs_cim009v010301p.pdf) capable\n[Node.js](https://nodejs.org/) [Express](https://expressjs.com/) application in order to retrieve and alter context\ndata. This removes the need to use the command-line to invoke cUrl commands.\n\nThe tutorial is mainly concerned with discussing code written in Node.js, however some of the results can be checked by\nmaking [cUrl](https://ec.haxx.se/) commands.\n\nThe `docker-compose` files for this tutorial can be found on GitHub: \n\n [FIWARE 603: Traversing Linked Data Programmatically](https://github.com/Fiware/tutorials.Working-with-Linked-Data)\n\n\n# Working with Linked Data Entities\n\n> - “This is the house that Jack built.\n> - This is the malt that lay in the house that Jack built.\n> - This is the rat that ate the malt
That lay in the house that Jack built.\n> - This is the cat
That killed the rat that ate the malt
That lay in the house that Jack built.\n> - This is the dog that chased the cat
That killed the rat that ate the malt
That lay in the house that\n> Jack built.”\n>\n> ― This Is the House That Jack Built, Traditional English Nursery Rhyme\n\nNSGI-LD is an evolution of NGSI-v2, so it should not be surprising that Smart solutions based on NSGI-LD will need to\ncover the same basic scenarios as outlined in the previous NGSI-v2\n[tutorial](https://github.com/FIWARE/tutorials.Accessing-Context/) on programatic data access.\n\nNGSI-LD Linked data formalizes the structure of context entities to a greater degree, through restricting data\nattributes to be defined as either _Property_ attributes or _Relationship_ attributes only. This means that it is\npossible to traverse the context data graph with greater certainty when moving from one _Relationship_ to another. All\nthe context data entities within the system are defined by JSON-LD data models, which are formally defined by\nreferencing a context file, and this programatic definition should guarantee that the associated linked entity exists.\n\nThree basic data access scenarios for the supermaket are defined below:\n\n- Reading Data - e.g. Give me all the data for the **Building** entity `urn:ngsi-ld:Building:store001`\n- Aggregation - e.g. Combine the **Products** entities sold in **Building** `urn:ngsi-ld:Building:store001` and\n display the goods for sale\n- Altering context within the system - e.g. Make a sale of a product:\n - Update the daily sales records by the price of the **Product**\n - decrement the `numberOfItems` of the **Shelf** entity\n - Create a new Transaction Log record showing the sale has occurred\n - Raise an alert in the warehouse if less than 10 objects remain on sale\n - etc.\n\nFurther advanced scenarios will be covered in later tutorials\n\n## Linked Data Entities within a stock management system\n\nThe supermarket data created in the [previous tutorial](https://github.com/FIWARE/tutorials.Relationships-Linked-Data/)\nwill be loaded into the context broker. The existing relationships between the entities are defined as shown below:\n\n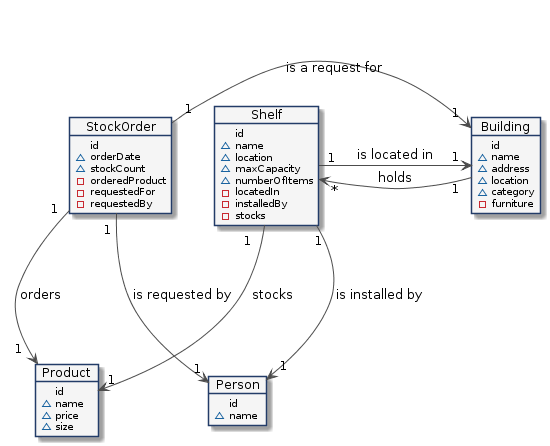\n\nThe **Building**, **Product**, **Shelf** and **StockOrder** entities will be used to display data on the frontend of our\ndemo application.\n\n## The teaching goal of this tutorial\n\nThe aim of this tutorial is to improve developer understanding of programmatic access of context data through defining\nand discussing a series of generic code examples covering common data access scenarios. For this purpose a simple\nNode.js Express application will be created.\n\nThe intention here is not to teach users how to write an application in Express - indeed any language could have been\nchosen. It is merely to show how **any** sample programming language could be used alter the context to achieve the\nbusiness logic goals.\n\nObviously, your choice of programming language will depend upon your own business needs - when reading the code below\nplease keep this in mind and substitute Node.js with your own programming language as appropriate.\n\n# Stock Management Frontend\n\nAll the code Node.js Express for the demo can be found within the `ngsi-ld` folder within the GitHub repository.\n[Stock Management example](https://github.com/FIWARE/tutorials.Step-by-Step/tree/master/context-provider). The\napplication runs on the following URLs:\n\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store001`\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store002`\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store003`\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store004`\n\n> :information_source: **Tip** Additionally, you can also watch the status of recent requests yourself by following the\n> container logs or viewing information on `localhost:3000/app/monitor` on a web browser.\n>\n> 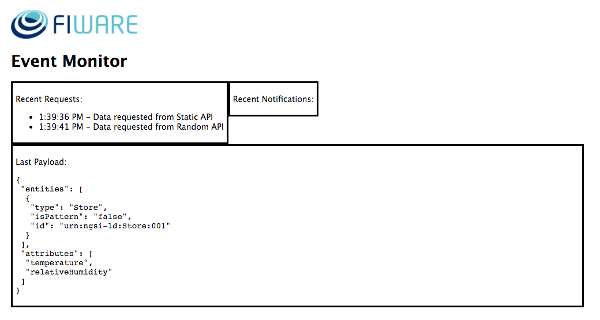\n\n# Prerequisites\n\n## Docker\n\nTo keep things simple all components will be run using [Docker](https://www.docker.com). **Docker** is a container\ntechnology which allows to different components isolated into their respective environments.\n\n- To install Docker on Windows follow the instructions [here](https://docs.docker.com/docker-for-windows/)\n- To install Docker on Mac follow the instructions [here](https://docs.docker.com/docker-for-mac/)\n- To install Docker on Linux follow the instructions [here](https://docs.docker.com/install/)\n\n**Docker Compose** is a tool for defining and running multi-container Docker applications. A\n[YAML file](https://raw.githubusercontent.com/fiware/tutorials.Relationships-Linked-Data/master/docker-compose.yml) is\nused configure the required services for the application. This means all container services can be brought up in a\nsingle command. Docker Compose is installed by default as part of Docker for Windows and Docker for Mac, however Linux\nusers will need to follow the instructions found [here](https://docs.docker.com/compose/install/)\n\n## Cygwin\n\nWe will start up our services using a simple bash script. Windows users should download [cygwin](http://www.cygwin.com/)\nto provide a command-line functionality similar to a Linux distribution on Windows.\n\n# Architecture\n\nThe demo Supermarket application will send and receive NGSI-LD calls to a compliant context broker. Since the NGSI-LD\ninterface is available on an experimental version of the\n[Orion Context Broker](https://fiware-orion.readthedocs.io/en/latest/), the demo application will only make use of one\nFIWARE component.\n\nCurrently, the Orion Context Broker relies on open source [MongoDB](https://www.mongodb.com/) technology to keep\npersistence of the context data it holds. To request context data from external sources, a simple Context Provider NGSI\nproxy has also been added. To visualize and interact with the Context we will add a simple Express application\n\nTherefore, the architecture will consist of three elements:\n\n- The [Orion Context Broker](https://fiware-orion.readthedocs.io/en/latest/) which will receive requests using\n [NGSI](https://fiware.github.io/specifications/OpenAPI/ngsiv2)\n- The underlying [MongoDB](https://www.mongodb.com/) database :\n - Used by the Orion Context Broker to hold context data information such as data entities, subscriptions and\n registrations\n- The **Stock Management Frontend** which will:\n - Display store information\n - Show which products can be bought at each store\n - Allow users to \"buy\" products and reduce the stock count.\n\nSince all interactions between the elements are initiated by HTTP requests, the entities can be containerized and run\nfrom exposed ports.\n\n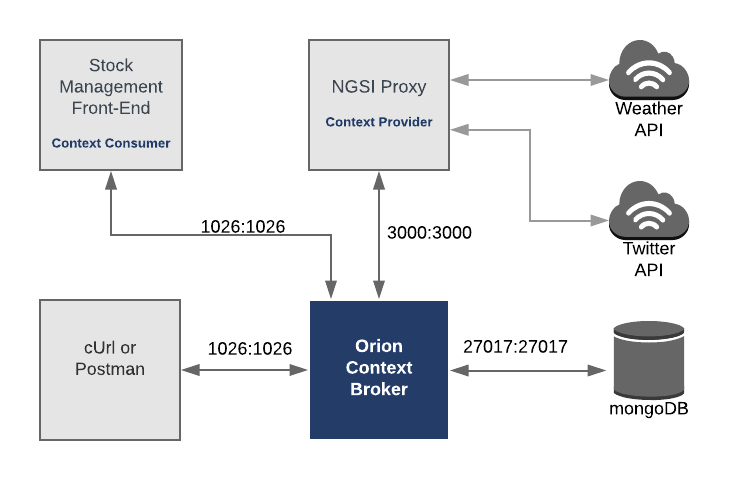\n\nThe necessary configuration information for the **Context Provider NGSI proxy** can be seen in the services section the\nof the associated `docker-compose.yml` file:\n\n```yaml\ntutorial:\n image: quay.io/fiware/tutorials.context-provider\n hostname: context-provider\n container_name: fiware-tutorial\n networks:\n - default\n expose:\n - \"3000\"\n ports:\n - \"3000:3000\"\n environment:\n - \"DEBUG=tutorial:*\"\n - \"WEB_APP_PORT=3000\"\n - \"NGSI_VERSION=ngsi-ld\"\n - \"CONTEXT_BROKER=http://orion:1026/ngsi-ld/v1\"\n```\n\nThe `tutorial` container is driven by environment variables as shown:\n\n| Key | Value | Description |\n| -------------- | ------------------------------ | ------------------------------------------------------------------------- |\n| DEBUG | `tutorial:*` | Debug flag used for logging |\n| WEB_APP_PORT | `3000` | Port used by the Context Provider NGSI proxy and web-app for viewing data |\n| CONTEXT_BROKER | `http://orion:1026/ngsi-ld/v1` | URL of the context broker to connect to update context |\n\nThe other `tutorial` container configuration values described in the YAML file are not used in this section of the\ntutorial.\n\nThe configuration information for MongoDB and the Orion Context Broker has been described in a\n[previous tutorial](https://github.com/FIWARE/tutorials.Relationships-Linked-Data/)\n\n# Start Up\n\nAll services can be initialised from the command-line by running the\n[services](https://github.com/FIWARE/tutorials.Relationships-Linked-Data/blob/master/services) Bash script provided\nwithin the repository. Please clone the repository and create the necessary images by running the commands as shown:\n\n```bash\ngit clone https://github.com/FIWARE/tutorials.Working-with-Linked-Data.git\ncd tutorials.Working-with-Linked-Data\n\n./services orion\n```\n\n> **Note:** If you want to clean up and start over again you can do so with the following command:\n>\n> ```\n> ./services stop\n> ```\n",
- "schema": "https://schema.getpostman.com/json/collection/v2.1.0/collection.json"
+ "name": "NGSI-LD Working with Linked Data",
+ "description": "[](https://github.com/FIWARE/catalogue/blob/master/core/README.md)\n[](https://www.etsi.org/deliver/etsi_gs/CIM/001_099/009/01.01.01_60/gs_CIM009v010101p.pdf)\n\nThis tutorial teaches FIWARE users how to architect and design a system based on **linked data** and to alter linked\ndata context programmatically. The tutorial extends the knowledge gained from the equivalent\n[NGSI-v2 tutorial](https://github.com/FIWARE/tutorials.Accessing-Context/) and enables a user understand how to write\ncode in an [NGSI-LD](https://www.etsi.org/deliver/etsi_gs/CIM/001_099/009/01.01.01_60/gs_CIM009v010101p.pdf) capable\n[Node.js](https://nodejs.org/) [Express](https://expressjs.com/) application in order to retrieve and alter context\ndata. This removes the need to use the command-line to invoke cUrl commands.\n\nThe tutorial is mainly concerned with discussing code written in Node.js, however some of the results can be checked by\nmaking [cUrl](https://ec.haxx.se/) commands.\n\nThe `docker-compose` files for this tutorial can be found on GitHub: \n\n [FIWARE 603: Traversing Linked Data Programmatically](https://github.com/Fiware/tutorials.Working-with-Linked-Data)\n\n\n# Working with Linked Data Entities\n\n> - “This is the house that Jack built.\n> - This is the malt that lay in the house that Jack built.\n> - This is the rat that ate the malt
That lay in the house that Jack built.\n> - This is the cat
That killed the rat that ate the malt
That lay in the house that Jack built.\n> - This is the dog that chased the cat
That killed the rat that ate the malt
That lay in the house that\n> Jack built.”\n>\n> ― This Is the House That Jack Built, Traditional English Nursery Rhyme\n\nNSGI-LD is an evolution of NGSI-v2, so it should not be surprising that Smart solutions based on NSGI-LD will need to\ncover the same basic scenarios as outlined in the previous NGSI-v2\n[tutorial](https://github.com/FIWARE/tutorials.Accessing-Context/) on programatic data access.\n\nNGSI-LD Linked data formalizes the structure of context entities to a greater degree, through restricting data\nattributes to be defined as either _Property_ attributes or _Relationship_ attributes only. This means that it is\npossible to traverse the context data graph with greater certainty when moving from one _Relationship_ to another. All\nthe context data entities within the system are defined by JSON-LD data models, which are formally defined by\nreferencing a context file, and this programatic definition should guarantee that the associated linked entity exists.\n\nThree basic data access scenarios for the supermaket are defined below:\n\n- Reading Data - e.g. Give me all the data for the **Building** entity `urn:ngsi-ld:Building:store001`\n- Aggregation - e.g. Combine the **Products** entities sold in **Building** `urn:ngsi-ld:Building:store001` and\n display the goods for sale\n- Altering context within the system - e.g. Make a sale of a product:\n - Update the daily sales records by the price of the **Product**\n - decrement the `numberOfItems` of the **Shelf** entity\n - Create a new Transaction Log record showing the sale has occurred\n - Raise an alert in the warehouse if less than 10 objects remain on sale\n - etc.\n\nFurther advanced scenarios will be covered in later tutorials\n\n## Linked Data Entities within a stock management system\n\nThe supermarket data created in the [previous tutorial](https://github.com/FIWARE/tutorials.Relationships-Linked-Data/)\nwill be loaded into the context broker. The existing relationships between the entities are defined as shown below:\n\n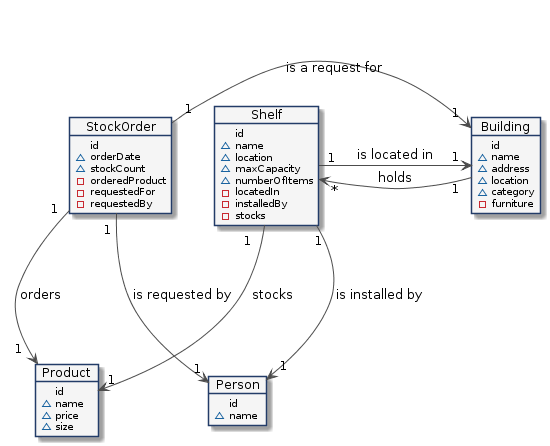\n\nThe **Building**, **Product**, **Shelf** and **StockOrder** entities will be used to display data on the frontend of our\ndemo application.\n\n## The teaching goal of this tutorial\n\nThe aim of this tutorial is to improve developer understanding of programmatic access of context data through defining\nand discussing a series of generic code examples covering common data access scenarios. For this purpose a simple\nNode.js Express application will be created.\n\nThe intention here is not to teach users how to write an application in Express - indeed any language could have been\nchosen. It is merely to show how **any** sample programming language could be used alter the context to achieve the\nbusiness logic goals.\n\nObviously, your choice of programming language will depend upon your own business needs - when reading the code below\nplease keep this in mind and substitute Node.js with your own programming language as appropriate.\n\n# Stock Management Frontend\n\nAll the code Node.js Express for the demo can be found within the `ngsi-ld` folder within the GitHub repository.\n[Stock Management example](https://github.com/FIWARE/tutorials.Step-by-Step/tree/master/context-provider). The\napplication runs on the following URLs:\n\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store001`\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store002`\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store003`\n- `http://localhost:3000/app/store/urn:ngsi-ld:Building:store004`\n\n> :information_source: **Tip** Additionally, you can also watch the status of recent requests yourself by following the\n> container logs or viewing information on `localhost:3000/app/monitor` on a web browser.\n>\n> 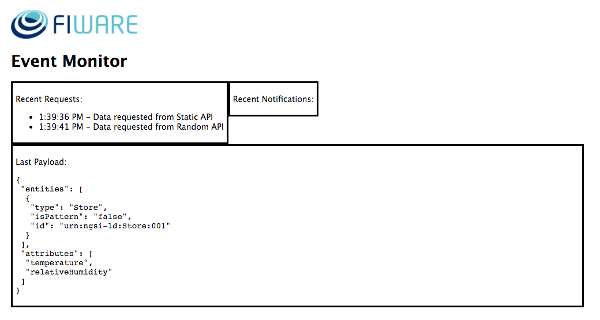\n\n# Prerequisites\n\n## Docker\n\nTo keep things simple all components will be run using [Docker](https://www.docker.com). **Docker** is a container\ntechnology which allows to different components isolated into their respective environments.\n\n- To install Docker on Windows follow the instructions [here](https://docs.docker.com/docker-for-windows/)\n- To install Docker on Mac follow the instructions [here](https://docs.docker.com/docker-for-mac/)\n- To install Docker on Linux follow the instructions [here](https://docs.docker.com/install/)\n\n**Docker Compose** is a tool for defining and running multi-container Docker applications. A\n[YAML file](https://raw.githubusercontent.com/fiware/tutorials.Relationships-Linked-Data/master/docker-compose.yml) is\nused configure the required services for the application. This means all container services can be brought up in a\nsingle command. Docker Compose is installed by default as part of Docker for Windows and Docker for Mac, however Linux\nusers will need to follow the instructions found [here](https://docs.docker.com/compose/install/)\n\n## Cygwin\n\nWe will start up our services using a simple bash script. Windows users should download [cygwin](http://www.cygwin.com/)\nto provide a command-line functionality similar to a Linux distribution on Windows.\n\n# Architecture\n\nThe demo Supermarket application will send and receive NGSI-LD calls to a compliant context broker. Since the NGSI-LD\ninterface is available on an experimental version of the\n[Orion Context Broker](https://fiware-orion.readthedocs.io/en/latest/), the demo application will only make use of one\nFIWARE component.\n\nCurrently, the Orion Context Broker relies on open source [MongoDB](https://www.mongodb.com/) technology to keep\npersistence of the context data it holds. To request context data from external sources, a simple Context Provider NGSI\nproxy has also been added. To visualize and interact with the Context we will add a simple Express application\n\nTherefore, the architecture will consist of three elements:\n\n- The [Orion Context Broker](https://fiware-orion.readthedocs.io/en/latest/) which will receive requests using\n [NGSI](https://fiware.github.io/specifications/OpenAPI/ngsiv2)\n- The underlying [MongoDB](https://www.mongodb.com/) database :\n - Used by the Orion Context Broker to hold context data information such as data entities, subscriptions and\n registrations\n- The **Stock Management Frontend** which will:\n - Display store information\n - Show which products can be bought at each store\n - Allow users to \"buy\" products and reduce the stock count.\n\nSince all interactions between the elements are initiated by HTTP requests, the entities can be containerized and run\nfrom exposed ports.\n\n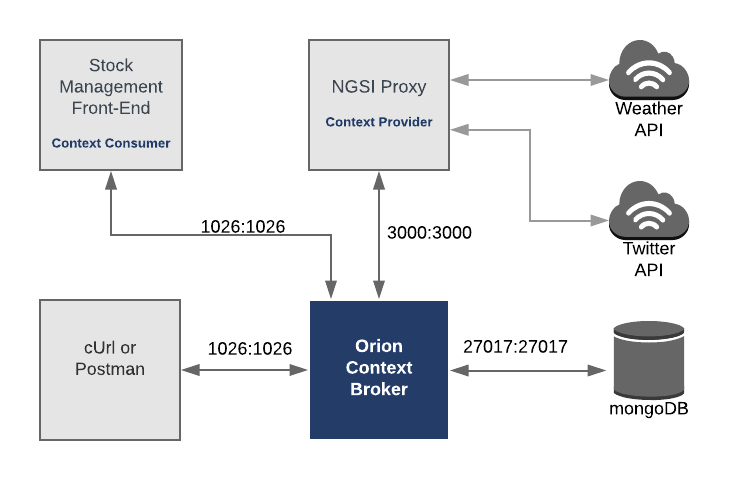\n\nThe necessary configuration information for the **Context Provider NGSI proxy** can be seen in the services section the\nof the associated `docker-compose.yml` file:\n\n```yaml\ntutorial:\n image: fiware/tutorials.context-provider\n hostname: context-provider\n container_name: fiware-tutorial\n networks:\n - default\n expose:\n - \"3000\"\n ports:\n - \"3000:3000\"\n environment:\n - \"DEBUG=tutorial:*\"\n - \"WEB_APP_PORT=3000\"\n - \"NGSI_VERSION=ngsi-ld\"\n - \"CONTEXT_BROKER=http://orion:1026/ngsi-ld/v1\"\n```\n\nThe `tutorial` container is driven by environment variables as shown:\n\n| Key | Value | Description |\n| -------------- | ------------------------------ | ------------------------------------------------------------------------- |\n| DEBUG | `tutorial:*` | Debug flag used for logging |\n| WEB_APP_PORT | `3000` | Port used by the Context Provider NGSI proxy and web-app for viewing data |\n| CONTEXT_BROKER | `http://orion:1026/ngsi-ld/v1` | URL of the context broker to connect to update context |\n\nThe other `tutorial` container configuration values described in the YAML file are not used in this section of the\ntutorial.\n\nThe configuration information for MongoDB and the Orion Context Broker has been described in a\n[previous tutorial](https://github.com/FIWARE/tutorials.Relationships-Linked-Data/)\n\n# Start Up\n\nAll services can be initialised from the command-line by running the\n[services](https://github.com/FIWARE/tutorials.Relationships-Linked-Data/blob/master/services) Bash script provided\nwithin the repository. Please clone the repository and create the necessary images by running the commands as shown:\n\n```bash\ngit clone https://github.com/FIWARE/tutorials.Working-with-Linked-Data.git\ncd tutorials.Working-with-Linked-Data\n\n./services orion\n```\n\n> **Note:** If you want to clean up and start over again you can do so with the following command:\n>\n> ```\n> ./services stop\n> ```\n",
+ "schema": "https://schema.getpostman.com/json/collection/v2.1.0/collection.json",
+ "_exporter_id": "513743",
+ "_collection_link": "https://fiware.postman.co/workspace/NGSI-v2-Tutorials~56ef8b2e-ab05-408c-bbe9-7714cfe08cf6/collection/513743-0bffb109-7af9-4ce3-b269-a53cb1e5c8e8?action=share&source=collection_link&creator=513743"
},
"item": [
{
@@ -20,13 +22,14 @@
"type": "text"
},
{
- "key": "Content-Type",
- "value": "application/ld+json",
- "type": "text"
+ "key": "",
+ "value": "",
+ "type": "text",
+ "disabled": true
}
],
"url": {
- "raw": "http://{{orion}}/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store001/?type=Building&options=keyValues",
+ "raw": "http://{{orion}}/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store001/?options=keyValues",
"protocol": "http",
"host": [
"{{orion}}"
@@ -39,10 +42,6 @@
""
],
"query": [
- {
- "key": "type",
- "value": "Building"
- },
{
"key": "options",
"value": "keyValues"
@@ -59,7 +58,6 @@
{
"listen": "prerequest",
"script": {
- "id": "f7057145-c2b9-4699-be04-77675a4bd430",
"type": "text/javascript",
"exec": [
""
@@ -69,15 +67,13 @@
{
"listen": "test",
"script": {
- "id": "05969c16-3c69-423d-87c6-6d5bb3e7fa27",
"type": "text/javascript",
"exec": [
""
]
}
}
- ],
- "protocolProfileBehavior": {}
+ ]
},
{
"name": "Aggregating and Traversing Linked Data",
@@ -93,13 +89,14 @@
"type": "text"
},
{
- "key": "Content-Type",
- "value": "application/ld+json",
- "type": "text"
+ "key": "",
+ "value": "",
+ "type": "text",
+ "disabled": true
}
],
"url": {
- "raw": "http://{{orion}}/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store001/?type=Building&options=keyValues&attrs=furniture",
+ "raw": "http://{{orion}}/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store001/?options=keyValues&attrs=furniture",
"protocol": "http",
"host": [
"{{orion}}"
@@ -112,10 +109,6 @@
""
],
"query": [
- {
- "key": "type",
- "value": "Building"
- },
{
"key": "options",
"value": "keyValues"
@@ -140,15 +133,16 @@
"value": "<{{datamodels-context.jsonld}}>; rel=\"http://www.w3.org/ns/json-ld#context\"; type=\"application/ld+json\"",
"type": "text"
},
- {
- "key": "Content-Type",
- "value": "application/ld+json",
- "type": "text"
- },
{
"key": "Accept",
"value": "application/json",
"type": "text"
+ },
+ {
+ "key": "",
+ "value": "",
+ "type": "text",
+ "disabled": true
}
],
"url": {
@@ -197,15 +191,16 @@
"type": "text"
},
{
- "key": "Content-Type",
- "value": "application/ld+json",
+ "key": "Accept",
+ "value": "application/json",
"type": "text"
},
{
- "key": "Accept",
- "value": "application/json",
+ "key": "",
+ "value": "",
"type": "text",
- "name": "Accept"
+ "name": "Accept",
+ "disabled": true
}
],
"url": {
@@ -244,8 +239,7 @@
"response": []
}
],
- "description": "To display information at the till, it is necessary to discover information about the products found within a Store.\nFrom the Data Entity diagram we can ascertain that:\n\n- **Building** entities hold related **Shelf** information within the `furniture` _Relationship_\n- **Shelf** entities hold related **Product** information within the `stocks` _Relationship_\n- Products hold `name` and `price` as _Property_ attributes of the **Product** entity itself.\n\nTherefore the code for the `displayTillInfo()` method will consist of the following steps.\n\n1. Make a request to the Context Broker to _find shelves within a known store_\n2. Reduce the result to a `id` parameter and make a second request to the Context Broker to _retrieve stocked products\n from shelves_\n3. Reduce the result to a `id` parameter and make a third request to the Context Broker to _retrieve product details for\n selected shelves_\n\nTo users familar with database joins, it may seem strange being forced to making a series of requests like this, however\nit is necessary due to scalability issues/concerns in a large distributed setup. Direct join requests are not possible\nwith NGSI-LD.",
- "protocolProfileBehavior": {}
+ "description": "To display information at the till, it is necessary to discover information about the products found within a Store.\nFrom the Data Entity diagram we can ascertain that:\n\n- **Building** entities hold related **Shelf** information within the `furniture` _Relationship_\n- **Shelf** entities hold related **Product** information within the `stocks` _Relationship_\n- Products hold `name` and `price` as _Property_ attributes of the **Product** entity itself.\n\nTherefore the code for the `displayTillInfo()` method will consist of the following steps.\n\n1. Make a request to the Context Broker to _find shelves within a known store_\n2. Reduce the result to a `id` parameter and make a second request to the Context Broker to _retrieve stocked products\n from shelves_\n3. Reduce the result to a `id` parameter and make a third request to the Context Broker to _retrieve product details for\n selected shelves_\n\nTo users familar with database joins, it may seem strange being forced to making a series of requests like this, however\nit is necessary due to scalability issues/concerns in a large distributed setup. Direct join requests are not possible\nwith NGSI-LD."
},
{
"name": "Updating Linked Data",
@@ -328,7 +322,7 @@
],
"body": {
"mode": "raw",
- "raw": "{ \"numberOfItems\": { \"type\": \"Property\", \"value\": 10 } }"
+ "raw": "{ \"numberOfItems\": { \"type\": \"Property\", \"value\": 9 } }"
},
"url": {
"raw": "http://{{orion}}/ngsi-ld/v1/entities/urn:ngsi-ld:Shelf:unit001/attrs",
@@ -348,8 +342,7 @@
},
"response": []
}
- ],
- "protocolProfileBehavior": {}
+ ]
},
{
"name": "Interoperability using Linked Data",
@@ -366,7 +359,7 @@
],
"body": {
"mode": "raw",
- "raw": "{\n \"id\": \"urn:ngsi-ld:Building:store005\",\n \"type\": \"ビル\",\n \"カテゴリー\": {\n \t\"type\": \"Property\",\n \"value\": [\"コマーシャル\"]\n },\n \"住所\": {\n \"type\": \"Property\",\n \"value\": {\n \"streetAddress\": \"Eisenacher Straße 98\",\n \"addressRegion\": \"Berlin\",\n \"addressLocality\": \"Marzahn\",\n \"postalCode\": \"12685\"\n }\n },\n \"場所\": {\n \"type\": \"GeoProperty\",\n \"value\": {\n \"type\": \"Point\",\n \"coordinates\": [13.5646, 52.5435]\n }\n },\n \"名前\": {\n \"type\": \"Property\",\n \"value\": \"Yuusui-en\"\n },\n \"@context\":\"https://fiware.github.io/tutorials.Step-by-Step/japanese-context.jsonld\"\n}"
+ "raw": "{\n \"id\": \"urn:ngsi-ld:Building:store005\",\n \"type\": \"ビル\",\n \"カテゴリー\": {\n \t\"type\": \"VocabularyProperty\",\n \"vocab\": [\"コマーシャル\"]\n },\n \"住所\": {\n \"type\": \"Property\",\n \"value\": {\n \"streetAddress\": \"Eisenacher Straße 98\",\n \"addressRegion\": \"Berlin\",\n \"addressLocality\": \"Marzahn\",\n \"postalCode\": \"12685\"\n }\n },\n \"場所\": {\n \"type\": \"GeoProperty\",\n \"value\": {\n \"type\": \"Point\",\n \"coordinates\": [13.5646, 52.5435]\n }\n },\n \"名前\": {\n \"type\": \"Property\",\n \"value\": \"Yuusui-en\"\n },\n \"@context\":\"http://context/japanese-user-context.jsonld\"\n}"
},
"url": {
"raw": "http://{{orion}}/ngsi-ld/v1/entities/",
@@ -392,7 +385,8 @@
"header": [
{
"key": "Content-Type",
- "value": "application/ld+json"
+ "value": "application/json",
+ "disabled": true
},
{
"key": "Link",
@@ -431,7 +425,8 @@
"header": [
{
"key": "Content-Type",
- "value": "application/ld+json"
+ "value": "application/ld+json",
+ "disabled": true
},
{
"key": "Link",
@@ -442,8 +437,7 @@
"key": "Accept",
"value": "application/json",
"type": "text",
- "name": "Accept",
- "disabled": true
+ "name": "Accept"
}
],
"url": {
@@ -475,18 +469,19 @@
},
{
"key": "Accept",
- "value": "application/json",
+ "value": "application/ld+json",
"type": "text",
"name": "Accept"
},
{
"key": "Link",
"value": "<{{japanese-context.jsonld}}>; rel=\"http://www.w3.org/ns/json-ld#context\"; type=\"application/ld+json\"",
- "type": "text"
+ "type": "text",
+ "disabled": true
}
],
"url": {
- "raw": "http://{{context-provider}}/japanese/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store005?",
+ "raw": "http://{{context-provider}}/japanese/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store005?options=keyValues",
"protocol": "http",
"host": [
"{{context-provider}}"
@@ -501,8 +496,7 @@
"query": [
{
"key": "options",
- "value": "keyValues",
- "disabled": true
+ "value": "keyValues"
}
]
},
@@ -516,7 +510,6 @@
{
"listen": "prerequest",
"script": {
- "id": "e93af0c5-33d3-4b9b-b5c5-675f2b6ec644",
"type": "text/javascript",
"exec": [
""
@@ -526,22 +519,19 @@
{
"listen": "test",
"script": {
- "id": "611eaae5-2a6a-41d8-a764-8c7b0ad917b9",
"type": "text/javascript",
"exec": [
""
]
}
}
- ],
- "protocolProfileBehavior": {}
+ ]
}
],
"event": [
{
"listen": "prerequest",
"script": {
- "id": "91cf54e0-d9c1-41af-81a4-50f33ee8fc8e",
"type": "text/javascript",
"exec": [
""
@@ -551,7 +541,6 @@
{
"listen": "test",
"script": {
- "id": "57f4bec4-173f-4e09-9032-08844d2c08be",
"type": "text/javascript",
"exec": [
""
@@ -561,35 +550,24 @@
],
"variable": [
{
- "id": "13ad13b0-7943-4168-819a-a210cb66f533",
"key": "orion",
- "value": "localhost:1026",
- "type": "string"
+ "value": "localhost:1026"
},
{
- "id": "3e295de3-71ad-45cf-ab2c-25d0937c5664",
"key": "datamodels-context.jsonld",
- "value": "https://fiware.github.io/tutorials.Step-by-Step/tutorials-context.jsonld",
- "type": "string"
+ "value": "http://context/user-context.jsonld"
},
{
- "id": "ca7b4061-b7ed-41da-82de-0f178dc905da",
"key": "device-edge",
- "value": "localhost:1027",
- "type": "string"
+ "value": "localhost:1027"
},
{
- "id": "860a0325-66c5-477d-a9db-877ecc2c20b1",
"key": "context-provider",
- "value": "localhost:3000",
- "type": "string"
+ "value": "localhost:3000"
},
{
- "id": "b37db20d-6a88-4f3f-b767-c941b7e6db94",
"key": "japanese-context.jsonld",
- "value": "https://fiware.github.io/tutorials.Step-by-Step/japanese-context.jsonld",
- "type": "string"
+ "value": "http://context/japanese-user-context.jsonld"
}
- ],
- "protocolProfileBehavior": {}
+ ]
}
\ No newline at end of file
diff --git a/README.md b/README.md
index 3f29696..e45b5f6 100644
--- a/README.md
+++ b/README.md
@@ -173,8 +173,9 @@ proxy has also been added. To visualize and interact with the Context we will ad
Therefore, the architecture will consist of three elements:
-- The [Orion Context Broker](https://fiware-orion.readthedocs.io/en/latest/) which will receive requests using
+- The [Context Broker](https://fiware-orion.readthedocs.io/en/latest/) which will receive requests using
[NGSI-LD](https://forge.etsi.org/swagger/ui/?url=https://forge.etsi.org/rep/NGSI-LD/NGSI-LD/raw/master/spec/updated/generated/full_api.json)
+- An HTTP **Web-Server** which offers static `@context` files defining the context entities within the system for both English and Japanese Users.
- The underlying [MongoDB](https://www.mongodb.com/) database :
- Used by the Orion Context Broker to hold context data information such as data entities, subscriptions and
registrations
@@ -229,7 +230,7 @@ All services can be initialised from the command-line by running the
[services](https://github.com/FIWARE/tutorials.Relationships-Linked-Data/blob/NGSI-v2/services) Bash script provided
within the repository. Please clone the repository and create the necessary images by running the commands as shown:
-```bash
+```console
git clone https://github.com/FIWARE/tutorials.Working-with-Linked-Data.git
cd tutorials.Working-with-Linked-Data
git checkout NGSI-v2
@@ -263,14 +264,14 @@ The code under discussion can be found within the `ngsi-ld/store` controller in
As usual, the code for HTTP access can be split out from the business logic of the Supermarket application itself. The
lower level calls have been placed into a library file, which simplifies the codebase. This needs to be included in the
header of the file as shown. Some constants are also required - for the Supermarket data, the `LinkHeader` is used to
-define location of the data models JSON-LD context as
-`https://fiware.github.io/tutorials.Step-by-Step/tutorials-context.jsonld`.
+define location of the data models JSON-LD context (which) as
+`http://context/ngsi-context.jsonld`.
```javascript
const ngsiLD = require('../../lib/ngsi-ld');
const LinkHeader =
- '; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json">';
+ '; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json">';
```
### Retrieve a known Store
@@ -336,7 +337,7 @@ The equivalent cUrl statement can be seen below:
```console
curl -G -X GET 'http://localhost:1026/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store001/' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
-H 'Content-Type: application/json' \
-d 'options=keyValues'
```
@@ -382,7 +383,7 @@ The equivalent cUrl statement can be seen below:
```console
curl -G -X GET 'http://localhost:1026/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store001/' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
-H 'Content-Type: application/json' \
-d 'options=keyValues' \
-d 'attrs=furniture' \
@@ -425,7 +426,7 @@ The equivalent cUrl statement can be seen below:
```console
curl -G -X GET 'http://localhost:1026/ngsi-ld/v1/entities/' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-d 'type=Shelf' \
@@ -469,7 +470,7 @@ The equivalent cUrl statement can be seen below:
```console
curl -G -X GET 'http://localhost:1026/ngsi-ld/v1/entities/' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-d 'type=Product' \
@@ -506,7 +507,7 @@ The equivalent cUrl statement can be seen below:
```console
curl -G -X GET 'http://localhost:1026/ngsi-ld/v1/entities/' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-d 'type=Shelf' \
@@ -545,7 +546,7 @@ The equivalent cUrl statement can be seen below:
```console
curl -X PATCH 'http://localhost:1026/ngsi-ld/v1/entities/urn:ngsi-ld:Shelf:unit001/attrs' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"' \
-H 'Content-Type: application/json' \
-d '{ "numberOfItems": { "type": "Property", "value": 10 } }'
```
@@ -560,21 +561,21 @@ As a demonstration of this, imagine we which to incorporate context data entitie
using a different schema. Rather than using `name`, `category`, `location` etc, our Japanese context provider is using
data attributes based on Kanji characters.
-The core NGSI-LD `@context` defines that `name` = `https://uri.etsi.org/ngsi-ld/name`, similarly we can define `名前` =
-`https://uri.etsi.org/ngsi-ld/name` and introduce alternate mappings for attribute names and enumerated values.
+The English user NGSI-LD `@context` defines that `name` = `https://schema.org/name`, similarly for a Japanese user we can define `名前` =
+`https://schema.org/name` and introduce alternate mappings for attribute names and enumerated values.
Provided that two systems can agree upon a **common** system of unique URIs for data interchange, they are free to
locally re-interpret those values within their own domain.
### Creating an Entity using an Alternate Schema
-An alternative Japanese JSON-LD `@context` file has been created and published to an external server. The file can be
-found here: `https://fiware.github.io/tutorials.Step-by-Step/japanese-context.jsonld`. Alternate data mappings can be
+An alternative Japanese JSON-LD `@context` file has been created and published to a web server. Within the docker network, the file can be
+found here: [`http://context/japanese-user-context.jsonld`](./data-models/japanese-user-context.jsonld). Alternate data mappings can be
found for all attribute names used within the tutorials.
> [!NOTE]
> For comparison the standard tutorial JSON-LD `@context` file can be found here:
-> `https://fiware.github.io/tutorials.Step-by-Step/tutorials-context.jsonld`
+> [`http://context/user-context.jsonld`](./data-models/user-context.jsonld)
#### 1️⃣ Request:
@@ -595,7 +596,10 @@ curl -L -X POST 'http://localhost:1026/ngsi-ld/v1/entities/' \
--data-raw '{
"id": "urn:ngsi-ld:Building:store005",
"type": "ビル",
- "カテゴリー": {"type": "Property", "value": ["コマーシャル"]},
+ "カテゴリー": {
+ "type": "VocabularyProperty",
+ "vocab": ["コマーシャル"]
+ },
"住所": {
"type": "Property",
"value": {
@@ -607,15 +611,21 @@ curl -L -X POST 'http://localhost:1026/ngsi-ld/v1/entities/' \
},
"場所": {
"type": "GeoProperty",
- "value": {"type": "Point","coordinates": [13.5646, 52.5435]}
+ "value": {
+ "type": "Point",
+ "coordinates": [13.5646, 52.5435]
+ }
+ },
+ "名前": {
+ "type": "Property",
+ "value": "Yuusui-en"
},
- "名前": {"type": "Property","value": "Yuusui-en"},
- "@context":"https://fiware.github.io/tutorials.Step-by-Step/japanese-context.jsonld"
+ "@context":"http://context/japanese-user-context.jsonld"
}'
```
-Note that in this example the name and address have been supplied as simple strings - JSON-LD does support an `@lang`
-definition to allow for internationalization, but this is an advanced topic which will not be discussed here.
+Note that in this example the name and address have been supplied as simple strings - JSON-LD does support a **LanguageProperty** `@lang`
+definition to allow for internationalization, but this is an advanced topic which will not be discussed here. Note that the `category`/ `カテゴリー` has been defined using a **VocabularyProperty**, so that the enumerated value `commercial`/ `"コマーシャル` can also be amended via the `@context`.
### Reading an Entity using the default schema
@@ -624,7 +634,7 @@ different attribute short names, the Japanese JSON-LD `@context` file agrees wit
the full URIs used for a **Building** entity - effectively it is using the same data model.
Therefore it is possible to request the new **Building** (created using the Japanese data model) and have it return
-using the short names specified in the standard tutorial JSON-LD `@context`, this is done by supplying the `Link` header
+using the short names specified in the standard Englisu User JSON-LD `@context`, this is done by supplying the `Link` header
is pointing to the tutorial JSON-LD `@context` file.
#### 2️⃣ Request:
@@ -632,7 +642,7 @@ is pointing to the tutorial JSON-LD `@context` file.
```console
curl -L -X GET 'http://localhost:1026/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store005' \
-H 'Content-Type: application/json' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"'
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"'
```
#### Response:
@@ -640,9 +650,9 @@ curl -L -X GET 'http://localhost:1026/ngsi-ld/v1/entities/urn:ngsi-ld:Building:s
The response is an ordinary **Building** entity which standard attribute names (such as `name` and `location` and it
also returns the standard enumeration for **Building** `category`.
-```jsonld
+```json
{
- "@context": "https://fiware.github.io/tutorials.Step-by-Step/tutorials-context.jsonld",
+ "@context": "http://context/ngsi-context.jsonld",
"id": "urn:ngsi-ld:Building:store005",
"type": "Building",
"address": {
@@ -659,7 +669,7 @@ also returns the standard enumeration for **Building** `category`.
"value": { "type": "Point", "coordinates": [13.5646, 52.5435] }
},
"name": { "type": "Property", "value": "Yuusui-en" },
- "category": { "type": "Property", "value": "commercial" }
+ "category": { "type": "VocabularyProperty", "vocab": "commercial" }
}
```
@@ -681,24 +691,25 @@ supplied in the `Link` header.
```console
curl -L -X GET 'http://localhost:1026/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store003' \
-H 'Content-Type: application/json' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"'
+-H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"'
```
#### Response:
The response is mixed - it uses attribute names and enumerations defined in `japanese-context.jsonld` with some
exceptions. NGSI-LD **is not** JSON-LD, in that the core context is always applied after the contexts received in the
-`Link` header. Since `name` and `location` are reserved attribute names, they are supplied using the default core
+`Link` header. Since `location` is a reserved attribute name, it is always supplied using the default core
context.
-```jsonld
+```json
{
- "@context": "https://fiware.github.io/tutorials.Step-by-Step/japanese-context.jsonld",
"id": "urn:ngsi-ld:Building:store003",
"type": "ビル",
- "家具": {
- "type": "Relationship",
- "object": ["urn:ngsi-ld:Shelf:unit006", "urn:ngsi-ld:Shelf:unit007", "urn:ngsi-ld:Shelf:unit008"]
+ "カテゴリー": {
+ "type": "VocabularyProperty",
+ "vocab": [
+ "コマーシャル"
+ ]
},
"住所": {
"type": "Property",
@@ -708,13 +719,32 @@ context.
"addressLocality": "Friedrichshain",
"postalCode": "10243"
},
- "検証済み": { "type": "Property", "value": false }
+ "検証済み": {
+ "type": "Property",
+ "value": false
+ }
},
- "name": { "type": "Property", "value": "East Side Galleria" },
- "カテゴリー": { "type": "Property", "value": "コマーシャル" },
"location": {
"type": "GeoProperty",
- "value": { "type": "Point", "coordinates": [13.4447, 52.5031] }
+ "value": {
+ "type": "Point",
+ "coordinates": [
+ 13.4447,
+ 52.5031
+ ]
+ }
+ },
+ "名前": {
+ "type": "Property",
+ "value": "East Side Galleria"
+ },
+ "家具": {
+ "type": "Relationship",
+ "object": [
+ "urn:ngsi-ld:Shelf:unit006",
+ "urn:ngsi-ld:Shelf:unit007",
+ "urn:ngsi-ld:Shelf:unit008"
+ ]
}
}
```
@@ -762,8 +792,7 @@ expansion/compaction operation.
```console
curl -L -X GET 'http://localhost:3000/japanese/ngsi-ld/v1/entities/urn:ngsi-ld:Building:store005' \
--H 'Accept: application/json' \
--H 'Link: ; rel="http://www.w3.org/ns/json-ld#context"; type="application/ld+json"'
+-H 'Accept: application/ld+json'
```
#### Response:
@@ -778,24 +807,57 @@ payload before sending data to the context broker.
{
"識別子": "urn:ngsi-ld:Building:store005",
"タイプ": "ビル",
- "カテゴリー": { "タイプ": "プロパティ", "値": "コマーシャル" },
"住所": {
- "タイプ": "プロパティ",
- "値": {
- "addressLocality": "Marzahn",
- "addressRegion": "Berlin",
- "postalCode": "12685",
- "streetAddress": "Eisenacher Straße 98"
- }
+ "addressLocality": "Marzahn",
+ "addressRegion": "Berlin",
+ "postalCode": "12685",
+ "streetAddress": "Eisenacher Straße 98"
},
+ "名前": "Yuusui-en",
"場所": {
- "タイプ": "ジオプロパティ",
- "値": { "タイプ": "Point", "座標": [13.5646, 52.5435] }
+ "タイプ": "場",
+ "座標": [
+ 13.5646,
+ 52.5435
+ ]
},
- "名前": { "タイプ": "プロパティ", "値": "Yuusui-en" }
+ "カテゴリー": {
+ "語彙": "コマーシャル"
+ }
+}
+```
+
+
+It is worth noting that the subattributes of `address` / `住所"` were left undefined by the `@context` files and therefore have remained unconverted. Both `address` and `住所"` are mapped to the same URI `http://schema.org/address`, it would have been possible to
+include additional mappings for the subattributes using the schema.org ontology as shown:
+
+```json
+{
+ "@context": {
+ "addressLocality": "http://schema.org/addressLocality",
+ "addressRegion": "http://schema.org/addressRegion",
+ "postalCode": "http://schema.org/postalCode",
+ "streetAddress": "http://schema.org/streetAddress"
+ }
}
+
+```
+
+and their equivalents in Japanese:
+
+```json
+{
+ "@context": {
+ "地方": "http://schema.org/addressLocality",
+ "地域": "http://schema.org/addressRegion",
+ "郵便番号": "http://schema.org/postalCode",
+ "住所": "http://schema.org/streetAddress"
+ }
+}
+
```
+
#### :arrow_forward: Video: JSON-LD Compaction & Expansion
[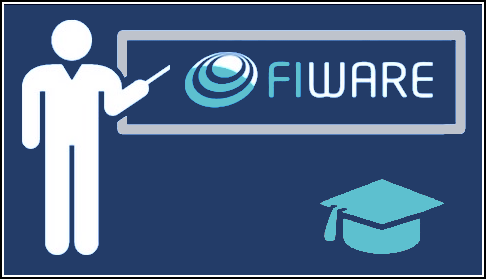](https://www.youtube.com/watch?v=Tm3fD89dqRE 'JSON-LD Compaction & Expansion')
diff --git a/conf/mime.types b/conf/mime.types
new file mode 100644
index 0000000..1d29c46
--- /dev/null
+++ b/conf/mime.types
@@ -0,0 +1,1858 @@
+# This file maps Internet media types to unique file extension(s).
+# Although created for httpd, this file is used by many software systems
+# and has been placed in the public domain for unlimited redisribution.
+#
+# The table below contains both registered and (common) unregistered types.
+# A type that has no unique extension can be ignored -- they are listed
+# here to guide configurations toward known types and to make it easier to
+# identify "new" types. File extensions are also commonly used to indicate
+# content languages and encodings, so choose them carefully.
+#
+# Internet media types should be registered as described in RFC 4288.
+# The registry is at .
+#
+# MIME type (lowercased) Extensions
+# ============================================ ==========
+# application/1d-interleaved-parityfec
+# application/3gpdash-qoe-report+xml
+# application/3gpp-ims+xml
+# application/a2l
+# application/activemessage
+# application/alto-costmap+json
+# application/alto-costmapfilter+json
+# application/alto-directory+json
+# application/alto-endpointcost+json
+# application/alto-endpointcostparams+json
+# application/alto-endpointprop+json
+# application/alto-endpointpropparams+json
+# application/alto-error+json
+# application/alto-networkmap+json
+# application/alto-networkmapfilter+json
+# application/aml
+application/andrew-inset ez
+# application/applefile
+application/applixware aw
+# application/atf
+# application/atfx
+application/atom+xml atom
+application/atomcat+xml atomcat
+# application/atomdeleted+xml
+# application/atomicmail
+application/atomsvc+xml atomsvc
+# application/atxml
+# application/auth-policy+xml
+# application/bacnet-xdd+zip
+# application/batch-smtp
+# application/beep+xml
+# application/calendar+json
+# application/calendar+xml
+# application/call-completion
+# application/cals-1840
+# application/cbor
+# application/ccmp+xml
+application/ccxml+xml ccxml
+# application/cdfx+xml
+application/cdmi-capability cdmia
+application/cdmi-container cdmic
+application/cdmi-domain cdmid
+application/cdmi-object cdmio
+application/cdmi-queue cdmiq
+# application/cdni
+# application/cea
+# application/cea-2018+xml
+# application/cellml+xml
+# application/cfw
+# application/cms
+# application/cnrp+xml
+# application/coap-group+json
+# application/commonground
+# application/conference-info+xml
+# application/cpl+xml
+# application/csrattrs
+# application/csta+xml
+# application/cstadata+xml
+# application/csvm+json
+application/cu-seeme cu
+# application/cybercash
+# application/dash+xml
+# application/dashdelta
+application/davmount+xml davmount
+# application/dca-rft
+# application/dcd
+# application/dec-dx
+# application/dialog-info+xml
+# application/dicom
+# application/dii
+# application/dit
+# application/dns
+application/docbook+xml dbk
+# application/dskpp+xml
+application/dssc+der dssc
+application/dssc+xml xdssc
+# application/dvcs
+application/ecmascript ecma
+# application/edi-consent
+# application/edi-x12
+# application/edifact
+# application/efi
+# application/emergencycalldata.comment+xml
+# application/emergencycalldata.deviceinfo+xml
+# application/emergencycalldata.providerinfo+xml
+# application/emergencycalldata.serviceinfo+xml
+# application/emergencycalldata.subscriberinfo+xml
+application/emma+xml emma
+# application/emotionml+xml
+# application/encaprtp
+# application/epp+xml
+application/epub+zip epub
+# application/eshop
+# application/example
+application/exi exi
+# application/fastinfoset
+# application/fastsoap
+# application/fdt+xml
+# application/fits
+application/font-tdpfr pfr
+# application/framework-attributes+xml
+# application/geo+json
+application/gml+xml gml
+application/gpx+xml gpx
+application/gxf gxf
+# application/gzip
+# application/h224
+# application/held+xml
+# application/http
+application/hyperstudio stk
+# application/ibe-key-request+xml
+# application/ibe-pkg-reply+xml
+# application/ibe-pp-data
+# application/iges
+# application/im-iscomposing+xml
+# application/index
+# application/index.cmd
+# application/index.obj
+# application/index.response
+# application/index.vnd
+application/inkml+xml ink inkml
+# application/iotp
+application/ipfix ipfix
+# application/ipp
+# application/isup
+# application/its+xml
+application/java-archive jar
+application/java-serialized-object ser
+application/java-vm class
+# application/javascript
+# application/jose
+# application/jose+json
+# application/jrd+json
+application/json json
+application/ld+json jsonld
+# application/json-patch+json
+# application/json-seq
+application/jsonml+json jsonml
+# application/jwk+json
+# application/jwk-set+json
+# application/jwt
+# application/kpml-request+xml
+# application/kpml-response+xml
+# application/ld+json
+# application/lgr+xml
+# application/link-format
+# application/load-control+xml
+application/lost+xml lostxml
+# application/lostsync+xml
+# application/lxf
+application/mac-binhex40 hqx
+application/mac-compactpro cpt
+# application/macwriteii
+application/mads+xml mads
+application/marc mrc
+application/marcxml+xml mrcx
+application/mathematica ma nb mb
+application/mathml+xml mathml
+# application/mathml-content+xml
+# application/mathml-presentation+xml
+# application/mbms-associated-procedure-description+xml
+# application/mbms-deregister+xml
+# application/mbms-envelope+xml
+# application/mbms-msk+xml
+# application/mbms-msk-response+xml
+# application/mbms-protection-description+xml
+# application/mbms-reception-report+xml
+# application/mbms-register+xml
+# application/mbms-register-response+xml
+# application/mbms-schedule+xml
+# application/mbms-user-service-description+xml
+application/mbox mbox
+# application/media-policy-dataset+xml
+# application/media_control+xml
+application/mediaservercontrol+xml mscml
+# application/merge-patch+json
+application/metalink+xml metalink
+application/metalink4+xml meta4
+application/mets+xml mets
+# application/mf4
+# application/mikey
+application/mods+xml mods
+# application/moss-keys
+# application/moss-signature
+# application/mosskey-data
+# application/mosskey-request
+application/mp21 m21 mp21
+application/mp4 mp4s
+# application/mpeg4-generic
+# application/mpeg4-iod
+# application/mpeg4-iod-xmt
+# application/mrb-consumer+xml
+# application/mrb-publish+xml
+# application/msc-ivr+xml
+# application/msc-mixer+xml
+application/msword doc dot
+application/mxf mxf
+# application/nasdata
+# application/news-checkgroups
+# application/news-groupinfo
+# application/news-transmission
+# application/nlsml+xml
+# application/nss
+# application/ocsp-request
+# application/ocsp-response
+application/octet-stream bin dms lrf mar so dist distz pkg bpk dump elc deploy
+application/oda oda
+# application/odx
+application/oebps-package+xml opf
+application/ogg ogx
+application/omdoc+xml omdoc
+application/onenote onetoc onetoc2 onetmp onepkg
+application/oxps oxps
+# application/p2p-overlay+xml
+# application/parityfec
+application/patch-ops-error+xml xer
+application/pdf pdf
+# application/pdx
+application/pgp-encrypted pgp
+# application/pgp-keys
+application/pgp-signature asc sig
+application/pics-rules prf
+# application/pidf+xml
+# application/pidf-diff+xml
+application/pkcs10 p10
+# application/pkcs12
+application/pkcs7-mime p7m p7c
+application/pkcs7-signature p7s
+application/pkcs8 p8
+application/pkix-attr-cert ac
+application/pkix-cert cer
+application/pkix-crl crl
+application/pkix-pkipath pkipath
+application/pkixcmp pki
+application/pls+xml pls
+# application/poc-settings+xml
+application/postscript ai eps ps
+# application/ppsp-tracker+json
+# application/problem+json
+# application/problem+xml
+# application/provenance+xml
+# application/prs.alvestrand.titrax-sheet
+application/prs.cww cww
+# application/prs.hpub+zip
+# application/prs.nprend
+# application/prs.plucker
+# application/prs.rdf-xml-crypt
+# application/prs.xsf+xml
+application/pskc+xml pskcxml
+# application/qsig
+# application/raptorfec
+# application/rdap+json
+application/rdf+xml rdf
+application/reginfo+xml rif
+application/relax-ng-compact-syntax rnc
+# application/remote-printing
+# application/reputon+json
+application/resource-lists+xml rl
+application/resource-lists-diff+xml rld
+# application/rfc+xml
+# application/riscos
+# application/rlmi+xml
+application/rls-services+xml rs
+application/rpki-ghostbusters gbr
+application/rpki-manifest mft
+application/rpki-roa roa
+# application/rpki-updown
+application/rsd+xml rsd
+application/rss+xml rss
+application/rtf rtf
+# application/rtploopback
+# application/rtx
+# application/samlassertion+xml
+# application/samlmetadata+xml
+application/sbml+xml sbml
+# application/scaip+xml
+# application/scim+json
+application/scvp-cv-request scq
+application/scvp-cv-response scs
+application/scvp-vp-request spq
+application/scvp-vp-response spp
+application/sdp sdp
+# application/sep+xml
+# application/sep-exi
+# application/session-info
+# application/set-payment
+application/set-payment-initiation setpay
+# application/set-registration
+application/set-registration-initiation setreg
+# application/sgml
+# application/sgml-open-catalog
+application/shf+xml shf
+# application/sieve
+# application/simple-filter+xml
+# application/simple-message-summary
+# application/simplesymbolcontainer
+# application/slate
+# application/smil
+application/smil+xml smi smil
+# application/smpte336m
+# application/soap+fastinfoset
+# application/soap+xml
+application/sparql-query rq
+application/sparql-results+xml srx
+# application/spirits-event+xml
+# application/sql
+application/srgs gram
+application/srgs+xml grxml
+application/sru+xml sru
+application/ssdl+xml ssdl
+application/ssml+xml ssml
+# application/tamp-apex-update
+# application/tamp-apex-update-confirm
+# application/tamp-community-update
+# application/tamp-community-update-confirm
+# application/tamp-error
+# application/tamp-sequence-adjust
+# application/tamp-sequence-adjust-confirm
+# application/tamp-status-query
+# application/tamp-status-response
+# application/tamp-update
+# application/tamp-update-confirm
+application/tei+xml tei teicorpus
+application/thraud+xml tfi
+# application/timestamp-query
+# application/timestamp-reply
+application/timestamped-data tsd
+# application/ttml+xml
+# application/tve-trigger
+# application/ulpfec
+# application/urc-grpsheet+xml
+# application/urc-ressheet+xml
+# application/urc-targetdesc+xml
+# application/urc-uisocketdesc+xml
+# application/vcard+json
+# application/vcard+xml
+# application/vemmi
+# application/vividence.scriptfile
+# application/vnd.3gpp-prose+xml
+# application/vnd.3gpp-prose-pc3ch+xml
+# application/vnd.3gpp.access-transfer-events+xml
+# application/vnd.3gpp.bsf+xml
+# application/vnd.3gpp.mid-call+xml
+application/vnd.3gpp.pic-bw-large plb
+application/vnd.3gpp.pic-bw-small psb
+application/vnd.3gpp.pic-bw-var pvb
+# application/vnd.3gpp.sms
+# application/vnd.3gpp.sms+xml
+# application/vnd.3gpp.srvcc-ext+xml
+# application/vnd.3gpp.srvcc-info+xml
+# application/vnd.3gpp.state-and-event-info+xml
+# application/vnd.3gpp.ussd+xml
+# application/vnd.3gpp2.bcmcsinfo+xml
+# application/vnd.3gpp2.sms
+application/vnd.3gpp2.tcap tcap
+# application/vnd.3lightssoftware.imagescal
+application/vnd.3m.post-it-notes pwn
+application/vnd.accpac.simply.aso aso
+application/vnd.accpac.simply.imp imp
+application/vnd.acucobol acu
+application/vnd.acucorp atc acutc
+application/vnd.adobe.air-application-installer-package+zip air
+# application/vnd.adobe.flash.movie
+application/vnd.adobe.formscentral.fcdt fcdt
+application/vnd.adobe.fxp fxp fxpl
+# application/vnd.adobe.partial-upload
+application/vnd.adobe.xdp+xml xdp
+application/vnd.adobe.xfdf xfdf
+# application/vnd.aether.imp
+# application/vnd.ah-barcode
+application/vnd.ahead.space ahead
+application/vnd.airzip.filesecure.azf azf
+application/vnd.airzip.filesecure.azs azs
+application/vnd.amazon.ebook azw
+# application/vnd.amazon.mobi8-ebook
+application/vnd.americandynamics.acc acc
+application/vnd.amiga.ami ami
+# application/vnd.amundsen.maze+xml
+application/vnd.android.package-archive apk
+# application/vnd.anki
+application/vnd.anser-web-certificate-issue-initiation cii
+application/vnd.anser-web-funds-transfer-initiation fti
+application/vnd.antix.game-component atx
+# application/vnd.apache.thrift.binary
+# application/vnd.apache.thrift.compact
+# application/vnd.apache.thrift.json
+# application/vnd.api+json
+application/vnd.apple.installer+xml mpkg
+application/vnd.apple.mpegurl m3u8
+# application/vnd.arastra.swi
+application/vnd.aristanetworks.swi swi
+# application/vnd.artsquare
+application/vnd.astraea-software.iota iota
+application/vnd.audiograph aep
+# application/vnd.autopackage
+# application/vnd.avistar+xml
+# application/vnd.balsamiq.bmml+xml
+# application/vnd.balsamiq.bmpr
+# application/vnd.bekitzur-stech+json
+# application/vnd.biopax.rdf+xml
+application/vnd.blueice.multipass mpm
+# application/vnd.bluetooth.ep.oob
+# application/vnd.bluetooth.le.oob
+application/vnd.bmi bmi
+application/vnd.businessobjects rep
+# application/vnd.cab-jscript
+# application/vnd.canon-cpdl
+# application/vnd.canon-lips
+# application/vnd.cendio.thinlinc.clientconf
+# application/vnd.century-systems.tcp_stream
+application/vnd.chemdraw+xml cdxml
+# application/vnd.chess-pgn
+application/vnd.chipnuts.karaoke-mmd mmd
+application/vnd.cinderella cdy
+# application/vnd.cirpack.isdn-ext
+# application/vnd.citationstyles.style+xml
+application/vnd.claymore cla
+application/vnd.cloanto.rp9 rp9
+application/vnd.clonk.c4group c4g c4d c4f c4p c4u
+application/vnd.cluetrust.cartomobile-config c11amc
+application/vnd.cluetrust.cartomobile-config-pkg c11amz
+# application/vnd.coffeescript
+# application/vnd.collection+json
+# application/vnd.collection.doc+json
+# application/vnd.collection.next+json
+# application/vnd.comicbook+zip
+# application/vnd.commerce-battelle
+application/vnd.commonspace csp
+application/vnd.contact.cmsg cdbcmsg
+# application/vnd.coreos.ignition+json
+application/vnd.cosmocaller cmc
+application/vnd.crick.clicker clkx
+application/vnd.crick.clicker.keyboard clkk
+application/vnd.crick.clicker.palette clkp
+application/vnd.crick.clicker.template clkt
+application/vnd.crick.clicker.wordbank clkw
+application/vnd.criticaltools.wbs+xml wbs
+application/vnd.ctc-posml pml
+# application/vnd.ctct.ws+xml
+# application/vnd.cups-pdf
+# application/vnd.cups-postscript
+application/vnd.cups-ppd ppd
+# application/vnd.cups-raster
+# application/vnd.cups-raw
+# application/vnd.curl
+application/vnd.curl.car car
+application/vnd.curl.pcurl pcurl
+# application/vnd.cyan.dean.root+xml
+# application/vnd.cybank
+application/vnd.dart dart
+application/vnd.data-vision.rdz rdz
+# application/vnd.debian.binary-package
+application/vnd.dece.data uvf uvvf uvd uvvd
+application/vnd.dece.ttml+xml uvt uvvt
+application/vnd.dece.unspecified uvx uvvx
+application/vnd.dece.zip uvz uvvz
+application/vnd.denovo.fcselayout-link fe_launch
+# application/vnd.desmume.movie
+# application/vnd.dir-bi.plate-dl-nosuffix
+# application/vnd.dm.delegation+xml
+application/vnd.dna dna
+# application/vnd.document+json
+application/vnd.dolby.mlp mlp
+# application/vnd.dolby.mobile.1
+# application/vnd.dolby.mobile.2
+# application/vnd.doremir.scorecloud-binary-document
+application/vnd.dpgraph dpg
+application/vnd.dreamfactory dfac
+# application/vnd.drive+json
+application/vnd.ds-keypoint kpxx
+# application/vnd.dtg.local
+# application/vnd.dtg.local.flash
+# application/vnd.dtg.local.html
+application/vnd.dvb.ait ait
+# application/vnd.dvb.dvbj
+# application/vnd.dvb.esgcontainer
+# application/vnd.dvb.ipdcdftnotifaccess
+# application/vnd.dvb.ipdcesgaccess
+# application/vnd.dvb.ipdcesgaccess2
+# application/vnd.dvb.ipdcesgpdd
+# application/vnd.dvb.ipdcroaming
+# application/vnd.dvb.iptv.alfec-base
+# application/vnd.dvb.iptv.alfec-enhancement
+# application/vnd.dvb.notif-aggregate-root+xml
+# application/vnd.dvb.notif-container+xml
+# application/vnd.dvb.notif-generic+xml
+# application/vnd.dvb.notif-ia-msglist+xml
+# application/vnd.dvb.notif-ia-registration-request+xml
+# application/vnd.dvb.notif-ia-registration-response+xml
+# application/vnd.dvb.notif-init+xml
+# application/vnd.dvb.pfr
+application/vnd.dvb.service svc
+# application/vnd.dxr
+application/vnd.dynageo geo
+# application/vnd.dzr
+# application/vnd.easykaraoke.cdgdownload
+# application/vnd.ecdis-update
+application/vnd.ecowin.chart mag
+# application/vnd.ecowin.filerequest
+# application/vnd.ecowin.fileupdate
+# application/vnd.ecowin.series
+# application/vnd.ecowin.seriesrequest
+# application/vnd.ecowin.seriesupdate
+# application/vnd.emclient.accessrequest+xml
+application/vnd.enliven nml
+# application/vnd.enphase.envoy
+# application/vnd.eprints.data+xml
+application/vnd.epson.esf esf
+application/vnd.epson.msf msf
+application/vnd.epson.quickanime qam
+application/vnd.epson.salt slt
+application/vnd.epson.ssf ssf
+# application/vnd.ericsson.quickcall
+application/vnd.eszigno3+xml es3 et3
+# application/vnd.etsi.aoc+xml
+# application/vnd.etsi.asic-e+zip
+# application/vnd.etsi.asic-s+zip
+# application/vnd.etsi.cug+xml
+# application/vnd.etsi.iptvcommand+xml
+# application/vnd.etsi.iptvdiscovery+xml
+# application/vnd.etsi.iptvprofile+xml
+# application/vnd.etsi.iptvsad-bc+xml
+# application/vnd.etsi.iptvsad-cod+xml
+# application/vnd.etsi.iptvsad-npvr+xml
+# application/vnd.etsi.iptvservice+xml
+# application/vnd.etsi.iptvsync+xml
+# application/vnd.etsi.iptvueprofile+xml
+# application/vnd.etsi.mcid+xml
+# application/vnd.etsi.mheg5
+# application/vnd.etsi.overload-control-policy-dataset+xml
+# application/vnd.etsi.pstn+xml
+# application/vnd.etsi.sci+xml
+# application/vnd.etsi.simservs+xml
+# application/vnd.etsi.timestamp-token
+# application/vnd.etsi.tsl+xml
+# application/vnd.etsi.tsl.der
+# application/vnd.eudora.data
+application/vnd.ezpix-album ez2
+application/vnd.ezpix-package ez3
+# application/vnd.f-secure.mobile
+# application/vnd.fastcopy-disk-image
+application/vnd.fdf fdf
+application/vnd.fdsn.mseed mseed
+application/vnd.fdsn.seed seed dataless
+# application/vnd.ffsns
+# application/vnd.filmit.zfc
+# application/vnd.fints
+# application/vnd.firemonkeys.cloudcell
+application/vnd.flographit gph
+application/vnd.fluxtime.clip ftc
+# application/vnd.font-fontforge-sfd
+application/vnd.framemaker fm frame maker book
+application/vnd.frogans.fnc fnc
+application/vnd.frogans.ltf ltf
+application/vnd.fsc.weblaunch fsc
+application/vnd.fujitsu.oasys oas
+application/vnd.fujitsu.oasys2 oa2
+application/vnd.fujitsu.oasys3 oa3
+application/vnd.fujitsu.oasysgp fg5
+application/vnd.fujitsu.oasysprs bh2
+# application/vnd.fujixerox.art-ex
+# application/vnd.fujixerox.art4
+application/vnd.fujixerox.ddd ddd
+application/vnd.fujixerox.docuworks xdw
+application/vnd.fujixerox.docuworks.binder xbd
+# application/vnd.fujixerox.docuworks.container
+# application/vnd.fujixerox.hbpl
+# application/vnd.fut-misnet
+application/vnd.fuzzysheet fzs
+application/vnd.genomatix.tuxedo txd
+# application/vnd.geo+json
+# application/vnd.geocube+xml
+application/vnd.geogebra.file ggb
+application/vnd.geogebra.slides ggs
+application/vnd.geogebra.tool ggt
+application/vnd.geometry-explorer gex gre
+application/vnd.geonext gxt
+application/vnd.geoplan g2w
+application/vnd.geospace g3w
+# application/vnd.gerber
+# application/vnd.globalplatform.card-content-mgt
+# application/vnd.globalplatform.card-content-mgt-response
+application/vnd.gmx gmx
+application/vnd.google-earth.kml+xml kml
+application/vnd.google-earth.kmz kmz
+# application/vnd.gov.sk.e-form+xml
+# application/vnd.gov.sk.e-form+zip
+# application/vnd.gov.sk.xmldatacontainer+xml
+application/vnd.grafeq gqf gqs
+# application/vnd.gridmp
+application/vnd.groove-account gac
+application/vnd.groove-help ghf
+application/vnd.groove-identity-message gim
+application/vnd.groove-injector grv
+application/vnd.groove-tool-message gtm
+application/vnd.groove-tool-template tpl
+application/vnd.groove-vcard vcg
+# application/vnd.hal+json
+application/vnd.hal+xml hal
+application/vnd.handheld-entertainment+xml zmm
+application/vnd.hbci hbci
+# application/vnd.hcl-bireports
+# application/vnd.hdt
+# application/vnd.heroku+json
+application/vnd.hhe.lesson-player les
+application/vnd.hp-hpgl hpgl
+application/vnd.hp-hpid hpid
+application/vnd.hp-hps hps
+application/vnd.hp-jlyt jlt
+application/vnd.hp-pcl pcl
+application/vnd.hp-pclxl pclxl
+# application/vnd.httphone
+application/vnd.hydrostatix.sof-data sfd-hdstx
+# application/vnd.hyperdrive+json
+# application/vnd.hzn-3d-crossword
+# application/vnd.ibm.afplinedata
+# application/vnd.ibm.electronic-media
+application/vnd.ibm.minipay mpy
+application/vnd.ibm.modcap afp listafp list3820
+application/vnd.ibm.rights-management irm
+application/vnd.ibm.secure-container sc
+application/vnd.iccprofile icc icm
+# application/vnd.ieee.1905
+application/vnd.igloader igl
+application/vnd.immervision-ivp ivp
+application/vnd.immervision-ivu ivu
+# application/vnd.ims.imsccv1p1
+# application/vnd.ims.imsccv1p2
+# application/vnd.ims.imsccv1p3
+# application/vnd.ims.lis.v2.result+json
+# application/vnd.ims.lti.v2.toolconsumerprofile+json
+# application/vnd.ims.lti.v2.toolproxy+json
+# application/vnd.ims.lti.v2.toolproxy.id+json
+# application/vnd.ims.lti.v2.toolsettings+json
+# application/vnd.ims.lti.v2.toolsettings.simple+json
+# application/vnd.informedcontrol.rms+xml
+# application/vnd.informix-visionary
+# application/vnd.infotech.project
+# application/vnd.infotech.project+xml
+# application/vnd.innopath.wamp.notification
+application/vnd.insors.igm igm
+application/vnd.intercon.formnet xpw xpx
+application/vnd.intergeo i2g
+# application/vnd.intertrust.digibox
+# application/vnd.intertrust.nncp
+application/vnd.intu.qbo qbo
+application/vnd.intu.qfx qfx
+# application/vnd.iptc.g2.catalogitem+xml
+# application/vnd.iptc.g2.conceptitem+xml
+# application/vnd.iptc.g2.knowledgeitem+xml
+# application/vnd.iptc.g2.newsitem+xml
+# application/vnd.iptc.g2.newsmessage+xml
+# application/vnd.iptc.g2.packageitem+xml
+# application/vnd.iptc.g2.planningitem+xml
+application/vnd.ipunplugged.rcprofile rcprofile
+application/vnd.irepository.package+xml irp
+application/vnd.is-xpr xpr
+application/vnd.isac.fcs fcs
+application/vnd.jam jam
+# application/vnd.japannet-directory-service
+# application/vnd.japannet-jpnstore-wakeup
+# application/vnd.japannet-payment-wakeup
+# application/vnd.japannet-registration
+# application/vnd.japannet-registration-wakeup
+# application/vnd.japannet-setstore-wakeup
+# application/vnd.japannet-verification
+# application/vnd.japannet-verification-wakeup
+application/vnd.jcp.javame.midlet-rms rms
+application/vnd.jisp jisp
+application/vnd.joost.joda-archive joda
+# application/vnd.jsk.isdn-ngn
+application/vnd.kahootz ktz ktr
+application/vnd.kde.karbon karbon
+application/vnd.kde.kchart chrt
+application/vnd.kde.kformula kfo
+application/vnd.kde.kivio flw
+application/vnd.kde.kontour kon
+application/vnd.kde.kpresenter kpr kpt
+application/vnd.kde.kspread ksp
+application/vnd.kde.kword kwd kwt
+application/vnd.kenameaapp htke
+application/vnd.kidspiration kia
+application/vnd.kinar kne knp
+application/vnd.koan skp skd skt skm
+application/vnd.kodak-descriptor sse
+application/vnd.las.las+xml lasxml
+# application/vnd.liberty-request+xml
+application/vnd.llamagraphics.life-balance.desktop lbd
+application/vnd.llamagraphics.life-balance.exchange+xml lbe
+application/vnd.lotus-1-2-3 123
+application/vnd.lotus-approach apr
+application/vnd.lotus-freelance pre
+application/vnd.lotus-notes nsf
+application/vnd.lotus-organizer org
+application/vnd.lotus-screencam scm
+application/vnd.lotus-wordpro lwp
+application/vnd.macports.portpkg portpkg
+# application/vnd.mapbox-vector-tile
+# application/vnd.marlin.drm.actiontoken+xml
+# application/vnd.marlin.drm.conftoken+xml
+# application/vnd.marlin.drm.license+xml
+# application/vnd.marlin.drm.mdcf
+# application/vnd.mason+json
+# application/vnd.maxmind.maxmind-db
+application/vnd.mcd mcd
+application/vnd.medcalcdata mc1
+application/vnd.mediastation.cdkey cdkey
+# application/vnd.meridian-slingshot
+application/vnd.mfer mwf
+application/vnd.mfmp mfm
+# application/vnd.micro+json
+application/vnd.micrografx.flo flo
+application/vnd.micrografx.igx igx
+# application/vnd.microsoft.portable-executable
+# application/vnd.miele+json
+application/vnd.mif mif
+# application/vnd.minisoft-hp3000-save
+# application/vnd.mitsubishi.misty-guard.trustweb
+application/vnd.mobius.daf daf
+application/vnd.mobius.dis dis
+application/vnd.mobius.mbk mbk
+application/vnd.mobius.mqy mqy
+application/vnd.mobius.msl msl
+application/vnd.mobius.plc plc
+application/vnd.mobius.txf txf
+application/vnd.mophun.application mpn
+application/vnd.mophun.certificate mpc
+# application/vnd.motorola.flexsuite
+# application/vnd.motorola.flexsuite.adsi
+# application/vnd.motorola.flexsuite.fis
+# application/vnd.motorola.flexsuite.gotap
+# application/vnd.motorola.flexsuite.kmr
+# application/vnd.motorola.flexsuite.ttc
+# application/vnd.motorola.flexsuite.wem
+# application/vnd.motorola.iprm
+application/vnd.mozilla.xul+xml xul
+# application/vnd.ms-3mfdocument
+application/vnd.ms-artgalry cil
+# application/vnd.ms-asf
+application/vnd.ms-cab-compressed cab
+# application/vnd.ms-color.iccprofile
+application/vnd.ms-excel xls xlm xla xlc xlt xlw
+application/vnd.ms-excel.addin.macroenabled.12 xlam
+application/vnd.ms-excel.sheet.binary.macroenabled.12 xlsb
+application/vnd.ms-excel.sheet.macroenabled.12 xlsm
+application/vnd.ms-excel.template.macroenabled.12 xltm
+application/vnd.ms-fontobject eot
+application/vnd.ms-htmlhelp chm
+application/vnd.ms-ims ims
+application/vnd.ms-lrm lrm
+# application/vnd.ms-office.activex+xml
+application/vnd.ms-officetheme thmx
+# application/vnd.ms-opentype
+# application/vnd.ms-package.obfuscated-opentype
+application/vnd.ms-pki.seccat cat
+application/vnd.ms-pki.stl stl
+# application/vnd.ms-playready.initiator+xml
+application/vnd.ms-powerpoint ppt pps pot
+application/vnd.ms-powerpoint.addin.macroenabled.12 ppam
+application/vnd.ms-powerpoint.presentation.macroenabled.12 pptm
+application/vnd.ms-powerpoint.slide.macroenabled.12 sldm
+application/vnd.ms-powerpoint.slideshow.macroenabled.12 ppsm
+application/vnd.ms-powerpoint.template.macroenabled.12 potm
+# application/vnd.ms-printdevicecapabilities+xml
+# application/vnd.ms-printing.printticket+xml
+# application/vnd.ms-printschematicket+xml
+application/vnd.ms-project mpp mpt
+# application/vnd.ms-tnef
+# application/vnd.ms-windows.devicepairing
+# application/vnd.ms-windows.nwprinting.oob
+# application/vnd.ms-windows.printerpairing
+# application/vnd.ms-windows.wsd.oob
+# application/vnd.ms-wmdrm.lic-chlg-req
+# application/vnd.ms-wmdrm.lic-resp
+# application/vnd.ms-wmdrm.meter-chlg-req
+# application/vnd.ms-wmdrm.meter-resp
+application/vnd.ms-word.document.macroenabled.12 docm
+application/vnd.ms-word.template.macroenabled.12 dotm
+application/vnd.ms-works wps wks wcm wdb
+application/vnd.ms-wpl wpl
+application/vnd.ms-xpsdocument xps
+# application/vnd.msa-disk-image
+application/vnd.mseq mseq
+# application/vnd.msign
+# application/vnd.multiad.creator
+# application/vnd.multiad.creator.cif
+# application/vnd.music-niff
+application/vnd.musician mus
+application/vnd.muvee.style msty
+application/vnd.mynfc taglet
+# application/vnd.ncd.control
+# application/vnd.ncd.reference
+# application/vnd.nervana
+# application/vnd.netfpx
+application/vnd.neurolanguage.nlu nlu
+# application/vnd.nintendo.nitro.rom
+# application/vnd.nintendo.snes.rom
+application/vnd.nitf ntf nitf
+application/vnd.noblenet-directory nnd
+application/vnd.noblenet-sealer nns
+application/vnd.noblenet-web nnw
+# application/vnd.nokia.catalogs
+# application/vnd.nokia.conml+wbxml
+# application/vnd.nokia.conml+xml
+# application/vnd.nokia.iptv.config+xml
+# application/vnd.nokia.isds-radio-presets
+# application/vnd.nokia.landmark+wbxml
+# application/vnd.nokia.landmark+xml
+# application/vnd.nokia.landmarkcollection+xml
+# application/vnd.nokia.n-gage.ac+xml
+application/vnd.nokia.n-gage.data ngdat
+application/vnd.nokia.n-gage.symbian.install n-gage
+# application/vnd.nokia.ncd
+# application/vnd.nokia.pcd+wbxml
+# application/vnd.nokia.pcd+xml
+application/vnd.nokia.radio-preset rpst
+application/vnd.nokia.radio-presets rpss
+application/vnd.novadigm.edm edm
+application/vnd.novadigm.edx edx
+application/vnd.novadigm.ext ext
+# application/vnd.ntt-local.content-share
+# application/vnd.ntt-local.file-transfer
+# application/vnd.ntt-local.ogw_remote-access
+# application/vnd.ntt-local.sip-ta_remote
+# application/vnd.ntt-local.sip-ta_tcp_stream
+application/vnd.oasis.opendocument.chart odc
+application/vnd.oasis.opendocument.chart-template otc
+application/vnd.oasis.opendocument.database odb
+application/vnd.oasis.opendocument.formula odf
+application/vnd.oasis.opendocument.formula-template odft
+application/vnd.oasis.opendocument.graphics odg
+application/vnd.oasis.opendocument.graphics-template otg
+application/vnd.oasis.opendocument.image odi
+application/vnd.oasis.opendocument.image-template oti
+application/vnd.oasis.opendocument.presentation odp
+application/vnd.oasis.opendocument.presentation-template otp
+application/vnd.oasis.opendocument.spreadsheet ods
+application/vnd.oasis.opendocument.spreadsheet-template ots
+application/vnd.oasis.opendocument.text odt
+application/vnd.oasis.opendocument.text-master odm
+application/vnd.oasis.opendocument.text-template ott
+application/vnd.oasis.opendocument.text-web oth
+# application/vnd.obn
+# application/vnd.oftn.l10n+json
+# application/vnd.oipf.contentaccessdownload+xml
+# application/vnd.oipf.contentaccessstreaming+xml
+# application/vnd.oipf.cspg-hexbinary
+# application/vnd.oipf.dae.svg+xml
+# application/vnd.oipf.dae.xhtml+xml
+# application/vnd.oipf.mippvcontrolmessage+xml
+# application/vnd.oipf.pae.gem
+# application/vnd.oipf.spdiscovery+xml
+# application/vnd.oipf.spdlist+xml
+# application/vnd.oipf.ueprofile+xml
+# application/vnd.oipf.userprofile+xml
+application/vnd.olpc-sugar xo
+# application/vnd.oma-scws-config
+# application/vnd.oma-scws-http-request
+# application/vnd.oma-scws-http-response
+# application/vnd.oma.bcast.associated-procedure-parameter+xml
+# application/vnd.oma.bcast.drm-trigger+xml
+# application/vnd.oma.bcast.imd+xml
+# application/vnd.oma.bcast.ltkm
+# application/vnd.oma.bcast.notification+xml
+# application/vnd.oma.bcast.provisioningtrigger
+# application/vnd.oma.bcast.sgboot
+# application/vnd.oma.bcast.sgdd+xml
+# application/vnd.oma.bcast.sgdu
+# application/vnd.oma.bcast.simple-symbol-container
+# application/vnd.oma.bcast.smartcard-trigger+xml
+# application/vnd.oma.bcast.sprov+xml
+# application/vnd.oma.bcast.stkm
+# application/vnd.oma.cab-address-book+xml
+# application/vnd.oma.cab-feature-handler+xml
+# application/vnd.oma.cab-pcc+xml
+# application/vnd.oma.cab-subs-invite+xml
+# application/vnd.oma.cab-user-prefs+xml
+# application/vnd.oma.dcd
+# application/vnd.oma.dcdc
+application/vnd.oma.dd2+xml dd2
+# application/vnd.oma.drm.risd+xml
+# application/vnd.oma.group-usage-list+xml
+# application/vnd.oma.lwm2m+json
+# application/vnd.oma.lwm2m+tlv
+# application/vnd.oma.pal+xml
+# application/vnd.oma.poc.detailed-progress-report+xml
+# application/vnd.oma.poc.final-report+xml
+# application/vnd.oma.poc.groups+xml
+# application/vnd.oma.poc.invocation-descriptor+xml
+# application/vnd.oma.poc.optimized-progress-report+xml
+# application/vnd.oma.push
+# application/vnd.oma.scidm.messages+xml
+# application/vnd.oma.xcap-directory+xml
+# application/vnd.omads-email+xml
+# application/vnd.omads-file+xml
+# application/vnd.omads-folder+xml
+# application/vnd.omaloc-supl-init
+# application/vnd.onepager
+# application/vnd.openblox.game+xml
+# application/vnd.openblox.game-binary
+# application/vnd.openeye.oeb
+application/vnd.openofficeorg.extension oxt
+# application/vnd.openxmlformats-officedocument.custom-properties+xml
+# application/vnd.openxmlformats-officedocument.customxmlproperties+xml
+# application/vnd.openxmlformats-officedocument.drawing+xml
+# application/vnd.openxmlformats-officedocument.drawingml.chart+xml
+# application/vnd.openxmlformats-officedocument.drawingml.chartshapes+xml
+# application/vnd.openxmlformats-officedocument.drawingml.diagramcolors+xml
+# application/vnd.openxmlformats-officedocument.drawingml.diagramdata+xml
+# application/vnd.openxmlformats-officedocument.drawingml.diagramlayout+xml
+# application/vnd.openxmlformats-officedocument.drawingml.diagramstyle+xml
+# application/vnd.openxmlformats-officedocument.extended-properties+xml
+# application/vnd.openxmlformats-officedocument.presentationml.commentauthors+xml
+# application/vnd.openxmlformats-officedocument.presentationml.comments+xml
+# application/vnd.openxmlformats-officedocument.presentationml.handoutmaster+xml
+# application/vnd.openxmlformats-officedocument.presentationml.notesmaster+xml
+# application/vnd.openxmlformats-officedocument.presentationml.notesslide+xml
+application/vnd.openxmlformats-officedocument.presentationml.presentation pptx
+# application/vnd.openxmlformats-officedocument.presentationml.presentation.main+xml
+# application/vnd.openxmlformats-officedocument.presentationml.presprops+xml
+application/vnd.openxmlformats-officedocument.presentationml.slide sldx
+# application/vnd.openxmlformats-officedocument.presentationml.slide+xml
+# application/vnd.openxmlformats-officedocument.presentationml.slidelayout+xml
+# application/vnd.openxmlformats-officedocument.presentationml.slidemaster+xml
+application/vnd.openxmlformats-officedocument.presentationml.slideshow ppsx
+# application/vnd.openxmlformats-officedocument.presentationml.slideshow.main+xml
+# application/vnd.openxmlformats-officedocument.presentationml.slideupdateinfo+xml
+# application/vnd.openxmlformats-officedocument.presentationml.tablestyles+xml
+# application/vnd.openxmlformats-officedocument.presentationml.tags+xml
+application/vnd.openxmlformats-officedocument.presentationml.template potx
+# application/vnd.openxmlformats-officedocument.presentationml.template.main+xml
+# application/vnd.openxmlformats-officedocument.presentationml.viewprops+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.calcchain+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.chartsheet+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.comments+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.connections+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.dialogsheet+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.externallink+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.pivotcachedefinition+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.pivotcacherecords+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.pivottable+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.querytable+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.revisionheaders+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.revisionlog+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.sharedstrings+xml
+application/vnd.openxmlformats-officedocument.spreadsheetml.sheet xlsx
+# application/vnd.openxmlformats-officedocument.spreadsheetml.sheet.main+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.sheetmetadata+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.styles+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.table+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.tablesinglecells+xml
+application/vnd.openxmlformats-officedocument.spreadsheetml.template xltx
+# application/vnd.openxmlformats-officedocument.spreadsheetml.template.main+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.usernames+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.volatiledependencies+xml
+# application/vnd.openxmlformats-officedocument.spreadsheetml.worksheet+xml
+# application/vnd.openxmlformats-officedocument.theme+xml
+# application/vnd.openxmlformats-officedocument.themeoverride+xml
+# application/vnd.openxmlformats-officedocument.vmldrawing
+# application/vnd.openxmlformats-officedocument.wordprocessingml.comments+xml
+application/vnd.openxmlformats-officedocument.wordprocessingml.document docx
+# application/vnd.openxmlformats-officedocument.wordprocessingml.document.glossary+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.document.main+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.endnotes+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.fonttable+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.footer+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.footnotes+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.numbering+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.settings+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.styles+xml
+application/vnd.openxmlformats-officedocument.wordprocessingml.template dotx
+# application/vnd.openxmlformats-officedocument.wordprocessingml.template.main+xml
+# application/vnd.openxmlformats-officedocument.wordprocessingml.websettings+xml
+# application/vnd.openxmlformats-package.core-properties+xml
+# application/vnd.openxmlformats-package.digital-signature-xmlsignature+xml
+# application/vnd.openxmlformats-package.relationships+xml
+# application/vnd.oracle.resource+json
+# application/vnd.orange.indata
+# application/vnd.osa.netdeploy
+application/vnd.osgeo.mapguide.package mgp
+# application/vnd.osgi.bundle
+application/vnd.osgi.dp dp
+application/vnd.osgi.subsystem esa
+# application/vnd.otps.ct-kip+xml
+# application/vnd.oxli.countgraph
+# application/vnd.pagerduty+json
+application/vnd.palm pdb pqa oprc
+# application/vnd.panoply
+# application/vnd.paos.xml
+application/vnd.pawaafile paw
+# application/vnd.pcos
+application/vnd.pg.format str
+application/vnd.pg.osasli ei6
+# application/vnd.piaccess.application-licence
+application/vnd.picsel efif
+application/vnd.pmi.widget wg
+# application/vnd.poc.group-advertisement+xml
+application/vnd.pocketlearn plf
+application/vnd.powerbuilder6 pbd
+# application/vnd.powerbuilder6-s
+# application/vnd.powerbuilder7
+# application/vnd.powerbuilder7-s
+# application/vnd.powerbuilder75
+# application/vnd.powerbuilder75-s
+# application/vnd.preminet
+application/vnd.previewsystems.box box
+application/vnd.proteus.magazine mgz
+application/vnd.publishare-delta-tree qps
+application/vnd.pvi.ptid1 ptid
+# application/vnd.pwg-multiplexed
+# application/vnd.pwg-xhtml-print+xml
+# application/vnd.qualcomm.brew-app-res
+# application/vnd.quarantainenet
+application/vnd.quark.quarkxpress qxd qxt qwd qwt qxl qxb
+# application/vnd.quobject-quoxdocument
+# application/vnd.radisys.moml+xml
+# application/vnd.radisys.msml+xml
+# application/vnd.radisys.msml-audit+xml
+# application/vnd.radisys.msml-audit-conf+xml
+# application/vnd.radisys.msml-audit-conn+xml
+# application/vnd.radisys.msml-audit-dialog+xml
+# application/vnd.radisys.msml-audit-stream+xml
+# application/vnd.radisys.msml-conf+xml
+# application/vnd.radisys.msml-dialog+xml
+# application/vnd.radisys.msml-dialog-base+xml
+# application/vnd.radisys.msml-dialog-fax-detect+xml
+# application/vnd.radisys.msml-dialog-fax-sendrecv+xml
+# application/vnd.radisys.msml-dialog-group+xml
+# application/vnd.radisys.msml-dialog-speech+xml
+# application/vnd.radisys.msml-dialog-transform+xml
+# application/vnd.rainstor.data
+# application/vnd.rapid
+# application/vnd.rar
+application/vnd.realvnc.bed bed
+application/vnd.recordare.musicxml mxl
+application/vnd.recordare.musicxml+xml musicxml
+# application/vnd.renlearn.rlprint
+application/vnd.rig.cryptonote cryptonote
+application/vnd.rim.cod cod
+application/vnd.rn-realmedia rm
+application/vnd.rn-realmedia-vbr rmvb
+application/vnd.route66.link66+xml link66
+# application/vnd.rs-274x
+# application/vnd.ruckus.download
+# application/vnd.s3sms
+application/vnd.sailingtracker.track st
+# application/vnd.sbm.cid
+# application/vnd.sbm.mid2
+# application/vnd.scribus
+# application/vnd.sealed.3df
+# application/vnd.sealed.csf
+# application/vnd.sealed.doc
+# application/vnd.sealed.eml
+# application/vnd.sealed.mht
+# application/vnd.sealed.net
+# application/vnd.sealed.ppt
+# application/vnd.sealed.tiff
+# application/vnd.sealed.xls
+# application/vnd.sealedmedia.softseal.html
+# application/vnd.sealedmedia.softseal.pdf
+application/vnd.seemail see
+application/vnd.sema sema
+application/vnd.semd semd
+application/vnd.semf semf
+application/vnd.shana.informed.formdata ifm
+application/vnd.shana.informed.formtemplate itp
+application/vnd.shana.informed.interchange iif
+application/vnd.shana.informed.package ipk
+application/vnd.simtech-mindmapper twd twds
+# application/vnd.siren+json
+application/vnd.smaf mmf
+# application/vnd.smart.notebook
+application/vnd.smart.teacher teacher
+# application/vnd.software602.filler.form+xml
+# application/vnd.software602.filler.form-xml-zip
+application/vnd.solent.sdkm+xml sdkm sdkd
+application/vnd.spotfire.dxp dxp
+application/vnd.spotfire.sfs sfs
+# application/vnd.sss-cod
+# application/vnd.sss-dtf
+# application/vnd.sss-ntf
+application/vnd.stardivision.calc sdc
+application/vnd.stardivision.draw sda
+application/vnd.stardivision.impress sdd
+application/vnd.stardivision.math smf
+application/vnd.stardivision.writer sdw vor
+application/vnd.stardivision.writer-global sgl
+application/vnd.stepmania.package smzip
+application/vnd.stepmania.stepchart sm
+# application/vnd.street-stream
+# application/vnd.sun.wadl+xml
+application/vnd.sun.xml.calc sxc
+application/vnd.sun.xml.calc.template stc
+application/vnd.sun.xml.draw sxd
+application/vnd.sun.xml.draw.template std
+application/vnd.sun.xml.impress sxi
+application/vnd.sun.xml.impress.template sti
+application/vnd.sun.xml.math sxm
+application/vnd.sun.xml.writer sxw
+application/vnd.sun.xml.writer.global sxg
+application/vnd.sun.xml.writer.template stw
+application/vnd.sus-calendar sus susp
+application/vnd.svd svd
+# application/vnd.swiftview-ics
+application/vnd.symbian.install sis sisx
+application/vnd.syncml+xml xsm
+application/vnd.syncml.dm+wbxml bdm
+application/vnd.syncml.dm+xml xdm
+# application/vnd.syncml.dm.notification
+# application/vnd.syncml.dmddf+wbxml
+# application/vnd.syncml.dmddf+xml
+# application/vnd.syncml.dmtnds+wbxml
+# application/vnd.syncml.dmtnds+xml
+# application/vnd.syncml.ds.notification
+application/vnd.tao.intent-module-archive tao
+application/vnd.tcpdump.pcap pcap cap dmp
+# application/vnd.tmd.mediaflex.api+xml
+# application/vnd.tml
+application/vnd.tmobile-livetv tmo
+application/vnd.trid.tpt tpt
+application/vnd.triscape.mxs mxs
+application/vnd.trueapp tra
+# application/vnd.truedoc
+# application/vnd.ubisoft.webplayer
+application/vnd.ufdl ufd ufdl
+application/vnd.uiq.theme utz
+application/vnd.umajin umj
+application/vnd.unity unityweb
+application/vnd.uoml+xml uoml
+# application/vnd.uplanet.alert
+# application/vnd.uplanet.alert-wbxml
+# application/vnd.uplanet.bearer-choice
+# application/vnd.uplanet.bearer-choice-wbxml
+# application/vnd.uplanet.cacheop
+# application/vnd.uplanet.cacheop-wbxml
+# application/vnd.uplanet.channel
+# application/vnd.uplanet.channel-wbxml
+# application/vnd.uplanet.list
+# application/vnd.uplanet.list-wbxml
+# application/vnd.uplanet.listcmd
+# application/vnd.uplanet.listcmd-wbxml
+# application/vnd.uplanet.signal
+# application/vnd.uri-map
+# application/vnd.valve.source.material
+application/vnd.vcx vcx
+# application/vnd.vd-study
+# application/vnd.vectorworks
+# application/vnd.vel+json
+# application/vnd.verimatrix.vcas
+# application/vnd.vidsoft.vidconference
+application/vnd.visio vsd vst vss vsw
+application/vnd.visionary vis
+# application/vnd.vividence.scriptfile
+application/vnd.vsf vsf
+# application/vnd.wap.sic
+# application/vnd.wap.slc
+application/vnd.wap.wbxml wbxml
+application/vnd.wap.wmlc wmlc
+application/vnd.wap.wmlscriptc wmlsc
+application/vnd.webturbo wtb
+# application/vnd.wfa.p2p
+# application/vnd.wfa.wsc
+# application/vnd.windows.devicepairing
+# application/vnd.wmc
+# application/vnd.wmf.bootstrap
+# application/vnd.wolfram.mathematica
+# application/vnd.wolfram.mathematica.package
+application/vnd.wolfram.player nbp
+application/vnd.wordperfect wpd
+application/vnd.wqd wqd
+# application/vnd.wrq-hp3000-labelled
+application/vnd.wt.stf stf
+# application/vnd.wv.csp+wbxml
+# application/vnd.wv.csp+xml
+# application/vnd.wv.ssp+xml
+# application/vnd.xacml+json
+application/vnd.xara xar
+application/vnd.xfdl xfdl
+# application/vnd.xfdl.webform
+# application/vnd.xmi+xml
+# application/vnd.xmpie.cpkg
+# application/vnd.xmpie.dpkg
+# application/vnd.xmpie.plan
+# application/vnd.xmpie.ppkg
+# application/vnd.xmpie.xlim
+application/vnd.yamaha.hv-dic hvd
+application/vnd.yamaha.hv-script hvs
+application/vnd.yamaha.hv-voice hvp
+application/vnd.yamaha.openscoreformat osf
+application/vnd.yamaha.openscoreformat.osfpvg+xml osfpvg
+# application/vnd.yamaha.remote-setup
+application/vnd.yamaha.smaf-audio saf
+application/vnd.yamaha.smaf-phrase spf
+# application/vnd.yamaha.through-ngn
+# application/vnd.yamaha.tunnel-udpencap
+# application/vnd.yaoweme
+application/vnd.yellowriver-custom-menu cmp
+application/vnd.zul zir zirz
+application/vnd.zzazz.deck+xml zaz
+application/voicexml+xml vxml
+# application/vq-rtcpxr
+application/wasm wasm
+# application/watcherinfo+xml
+# application/whoispp-query
+# application/whoispp-response
+application/widget wgt
+application/winhlp hlp
+# application/wita
+# application/wordperfect5.1
+application/wsdl+xml wsdl
+application/wspolicy+xml wspolicy
+application/x-7z-compressed 7z
+application/x-abiword abw
+application/x-ace-compressed ace
+# application/x-amf
+application/x-apple-diskimage dmg
+application/x-authorware-bin aab x32 u32 vox
+application/x-authorware-map aam
+application/x-authorware-seg aas
+application/x-bcpio bcpio
+application/x-bittorrent torrent
+application/x-blorb blb blorb
+application/x-bzip bz
+application/x-bzip2 bz2 boz
+application/x-cbr cbr cba cbt cbz cb7
+application/x-cdlink vcd
+application/x-cfs-compressed cfs
+application/x-chat chat
+application/x-chess-pgn pgn
+# application/x-compress
+application/x-conference nsc
+application/x-cpio cpio
+application/x-csh csh
+application/x-debian-package deb udeb
+application/x-dgc-compressed dgc
+application/x-director dir dcr dxr cst cct cxt w3d fgd swa
+application/x-doom wad
+application/x-dtbncx+xml ncx
+application/x-dtbook+xml dtb
+application/x-dtbresource+xml res
+application/x-dvi dvi
+application/x-envoy evy
+application/x-eva eva
+application/x-font-bdf bdf
+# application/x-font-dos
+# application/x-font-framemaker
+application/x-font-ghostscript gsf
+# application/x-font-libgrx
+application/x-font-linux-psf psf
+application/x-font-pcf pcf
+application/x-font-snf snf
+# application/x-font-speedo
+# application/x-font-sunos-news
+application/x-font-type1 pfa pfb pfm afm
+# application/x-font-vfont
+application/x-freearc arc
+application/x-futuresplash spl
+application/x-gca-compressed gca
+application/x-glulx ulx
+application/x-gnumeric gnumeric
+application/x-gramps-xml gramps
+application/x-gtar gtar
+# application/x-gzip
+application/x-hdf hdf
+application/x-install-instructions install
+application/x-iso9660-image iso
+application/x-java-jnlp-file jnlp
+application/x-latex latex
+application/x-lzh-compressed lzh lha
+application/x-mie mie
+application/x-mobipocket-ebook prc mobi
+application/x-ms-application application
+application/x-ms-shortcut lnk
+application/x-ms-wmd wmd
+application/x-ms-wmz wmz
+application/x-ms-xbap xbap
+application/x-msaccess mdb
+application/x-msbinder obd
+application/x-mscardfile crd
+application/x-msclip clp
+application/x-msdownload exe dll com bat msi
+application/x-msmediaview mvb m13 m14
+application/x-msmetafile wmf wmz emf emz
+application/x-msmoney mny
+application/x-mspublisher pub
+application/x-msschedule scd
+application/x-msterminal trm
+application/x-mswrite wri
+application/x-netcdf nc cdf
+application/x-nzb nzb
+application/x-pkcs12 p12 pfx
+application/x-pkcs7-certificates p7b spc
+application/x-pkcs7-certreqresp p7r
+application/x-rar-compressed rar
+application/x-research-info-systems ris
+application/x-sh sh
+application/x-shar shar
+application/x-shockwave-flash swf
+application/x-silverlight-app xap
+application/x-sql sql
+application/x-stuffit sit
+application/x-stuffitx sitx
+application/x-subrip srt
+application/x-sv4cpio sv4cpio
+application/x-sv4crc sv4crc
+application/x-t3vm-image t3
+application/x-tads gam
+application/x-tar tar
+application/x-tcl tcl
+application/x-tex tex
+application/x-tex-tfm tfm
+application/x-texinfo texinfo texi
+application/x-tgif obj
+application/x-ustar ustar
+application/x-wais-source src
+# application/x-www-form-urlencoded
+application/x-x509-ca-cert der crt
+application/x-xfig fig
+application/x-xliff+xml xlf
+application/x-xpinstall xpi
+application/x-xz xz
+application/x-zmachine z1 z2 z3 z4 z5 z6 z7 z8
+# application/x400-bp
+# application/xacml+xml
+application/xaml+xml xaml
+# application/xcap-att+xml
+# application/xcap-caps+xml
+application/xcap-diff+xml xdf
+# application/xcap-el+xml
+# application/xcap-error+xml
+# application/xcap-ns+xml
+# application/xcon-conference-info+xml
+# application/xcon-conference-info-diff+xml
+application/xenc+xml xenc
+application/xhtml+xml xhtml xht
+# application/xhtml-voice+xml
+application/xml xml xsl
+application/xml-dtd dtd
+# application/xml-external-parsed-entity
+# application/xml-patch+xml
+# application/xmpp+xml
+application/xop+xml xop
+application/xproc+xml xpl
+application/xslt+xml xslt
+application/xspf+xml xspf
+application/xv+xml mxml xhvml xvml xvm
+application/yang yang
+application/yin+xml yin
+application/zip zip
+# application/zlib
+# audio/1d-interleaved-parityfec
+# audio/32kadpcm
+# audio/3gpp
+# audio/3gpp2
+# audio/ac3
+audio/adpcm adp
+# audio/amr
+# audio/amr-wb
+# audio/amr-wb+
+# audio/aptx
+# audio/asc
+# audio/atrac-advanced-lossless
+# audio/atrac-x
+# audio/atrac3
+audio/basic au snd
+# audio/bv16
+# audio/bv32
+# audio/clearmode
+# audio/cn
+# audio/dat12
+# audio/dls
+# audio/dsr-es201108
+# audio/dsr-es202050
+# audio/dsr-es202211
+# audio/dsr-es202212
+# audio/dv
+# audio/dvi4
+# audio/eac3
+# audio/encaprtp
+# audio/evrc
+# audio/evrc-qcp
+# audio/evrc0
+# audio/evrc1
+# audio/evrcb
+# audio/evrcb0
+# audio/evrcb1
+# audio/evrcnw
+# audio/evrcnw0
+# audio/evrcnw1
+# audio/evrcwb
+# audio/evrcwb0
+# audio/evrcwb1
+# audio/evs
+# audio/example
+# audio/fwdred
+# audio/g711-0
+# audio/g719
+# audio/g722
+# audio/g7221
+# audio/g723
+# audio/g726-16
+# audio/g726-24
+# audio/g726-32
+# audio/g726-40
+# audio/g728
+# audio/g729
+# audio/g7291
+# audio/g729d
+# audio/g729e
+# audio/gsm
+# audio/gsm-efr
+# audio/gsm-hr-08
+# audio/ilbc
+# audio/ip-mr_v2.5
+# audio/isac
+# audio/l16
+# audio/l20
+# audio/l24
+# audio/l8
+# audio/lpc
+audio/midi mid midi kar rmi
+# audio/mobile-xmf
+audio/mp4 m4a mp4a
+# audio/mp4a-latm
+# audio/mpa
+# audio/mpa-robust
+audio/mpeg mpga mp2 mp2a mp3 m2a m3a
+# audio/mpeg4-generic
+# audio/musepack
+audio/ogg oga ogg spx opus
+# audio/opus
+# audio/parityfec
+# audio/pcma
+# audio/pcma-wb
+# audio/pcmu
+# audio/pcmu-wb
+# audio/prs.sid
+# audio/qcelp
+# audio/raptorfec
+# audio/red
+# audio/rtp-enc-aescm128
+# audio/rtp-midi
+# audio/rtploopback
+# audio/rtx
+audio/s3m s3m
+audio/silk sil
+# audio/smv
+# audio/smv-qcp
+# audio/smv0
+# audio/sp-midi
+# audio/speex
+# audio/t140c
+# audio/t38
+# audio/telephone-event
+# audio/tone
+# audio/uemclip
+# audio/ulpfec
+# audio/vdvi
+# audio/vmr-wb
+# audio/vnd.3gpp.iufp
+# audio/vnd.4sb
+# audio/vnd.audiokoz
+# audio/vnd.celp
+# audio/vnd.cisco.nse
+# audio/vnd.cmles.radio-events
+# audio/vnd.cns.anp1
+# audio/vnd.cns.inf1
+audio/vnd.dece.audio uva uvva
+audio/vnd.digital-winds eol
+# audio/vnd.dlna.adts
+# audio/vnd.dolby.heaac.1
+# audio/vnd.dolby.heaac.2
+# audio/vnd.dolby.mlp
+# audio/vnd.dolby.mps
+# audio/vnd.dolby.pl2
+# audio/vnd.dolby.pl2x
+# audio/vnd.dolby.pl2z
+# audio/vnd.dolby.pulse.1
+audio/vnd.dra dra
+audio/vnd.dts dts
+audio/vnd.dts.hd dtshd
+# audio/vnd.dvb.file
+# audio/vnd.everad.plj
+# audio/vnd.hns.audio
+audio/vnd.lucent.voice lvp
+audio/vnd.ms-playready.media.pya pya
+# audio/vnd.nokia.mobile-xmf
+# audio/vnd.nortel.vbk
+audio/vnd.nuera.ecelp4800 ecelp4800
+audio/vnd.nuera.ecelp7470 ecelp7470
+audio/vnd.nuera.ecelp9600 ecelp9600
+# audio/vnd.octel.sbc
+# audio/vnd.qcelp
+# audio/vnd.rhetorex.32kadpcm
+audio/vnd.rip rip
+# audio/vnd.sealedmedia.softseal.mpeg
+# audio/vnd.vmx.cvsd
+# audio/vorbis
+# audio/vorbis-config
+audio/webm weba
+audio/x-aac aac
+audio/x-aiff aif aiff aifc
+audio/x-caf caf
+audio/x-flac flac
+audio/x-matroska mka
+audio/x-mpegurl m3u
+audio/x-ms-wax wax
+audio/x-ms-wma wma
+audio/x-pn-realaudio ram ra
+audio/x-pn-realaudio-plugin rmp
+# audio/x-tta
+audio/x-wav wav
+audio/xm xm
+chemical/x-cdx cdx
+chemical/x-cif cif
+chemical/x-cmdf cmdf
+chemical/x-cml cml
+chemical/x-csml csml
+# chemical/x-pdb
+chemical/x-xyz xyz
+font/collection ttc
+font/otf otf
+# font/sfnt
+font/ttf ttf
+font/woff woff
+font/woff2 woff2
+image/bmp bmp
+image/cgm cgm
+# image/dicom-rle
+# image/emf
+# image/example
+# image/fits
+image/g3fax g3
+image/gif gif
+image/ief ief
+# image/jls
+# image/jp2
+image/jpeg jpeg jpg jpe
+# image/jpm
+# image/jpx
+image/ktx ktx
+# image/naplps
+image/png png
+image/prs.btif btif
+# image/prs.pti
+# image/pwg-raster
+image/sgi sgi
+image/svg+xml svg svgz
+# image/t38
+image/tiff tiff tif
+# image/tiff-fx
+image/vnd.adobe.photoshop psd
+# image/vnd.airzip.accelerator.azv
+# image/vnd.cns.inf2
+image/vnd.dece.graphic uvi uvvi uvg uvvg
+image/vnd.djvu djvu djv
+image/vnd.dvb.subtitle sub
+image/vnd.dwg dwg
+image/vnd.dxf dxf
+image/vnd.fastbidsheet fbs
+image/vnd.fpx fpx
+image/vnd.fst fst
+image/vnd.fujixerox.edmics-mmr mmr
+image/vnd.fujixerox.edmics-rlc rlc
+# image/vnd.globalgraphics.pgb
+# image/vnd.microsoft.icon
+# image/vnd.mix
+# image/vnd.mozilla.apng
+image/vnd.ms-modi mdi
+image/vnd.ms-photo wdp
+image/vnd.net-fpx npx
+# image/vnd.radiance
+# image/vnd.sealed.png
+# image/vnd.sealedmedia.softseal.gif
+# image/vnd.sealedmedia.softseal.jpg
+# image/vnd.svf
+# image/vnd.tencent.tap
+# image/vnd.valve.source.texture
+image/vnd.wap.wbmp wbmp
+image/vnd.xiff xif
+# image/vnd.zbrush.pcx
+image/webp webp
+# image/wmf
+image/x-3ds 3ds
+image/x-cmu-raster ras
+image/x-cmx cmx
+image/x-freehand fh fhc fh4 fh5 fh7
+image/x-icon ico
+image/x-mrsid-image sid
+image/x-pcx pcx
+image/x-pict pic pct
+image/x-portable-anymap pnm
+image/x-portable-bitmap pbm
+image/x-portable-graymap pgm
+image/x-portable-pixmap ppm
+image/x-rgb rgb
+image/x-tga tga
+image/x-xbitmap xbm
+image/x-xpixmap xpm
+image/x-xwindowdump xwd
+# message/cpim
+# message/delivery-status
+# message/disposition-notification
+# message/example
+# message/external-body
+# message/feedback-report
+# message/global
+# message/global-delivery-status
+# message/global-disposition-notification
+# message/global-headers
+# message/http
+# message/imdn+xml
+# message/news
+# message/partial
+message/rfc822 eml mime
+# message/s-http
+# message/sip
+# message/sipfrag
+# message/tracking-status
+# message/vnd.si.simp
+# message/vnd.wfa.wsc
+# model/example
+# model/gltf+json
+model/iges igs iges
+model/mesh msh mesh silo
+model/vnd.collada+xml dae
+model/vnd.dwf dwf
+# model/vnd.flatland.3dml
+model/vnd.gdl gdl
+# model/vnd.gs-gdl
+# model/vnd.gs.gdl
+model/vnd.gtw gtw
+# model/vnd.moml+xml
+model/vnd.mts mts
+# model/vnd.opengex
+# model/vnd.parasolid.transmit.binary
+# model/vnd.parasolid.transmit.text
+# model/vnd.rosette.annotated-data-model
+# model/vnd.valve.source.compiled-map
+model/vnd.vtu vtu
+model/vrml wrl vrml
+model/x3d+binary x3db x3dbz
+# model/x3d+fastinfoset
+model/x3d+vrml x3dv x3dvz
+model/x3d+xml x3d x3dz
+# model/x3d-vrml
+# multipart/alternative
+# multipart/appledouble
+# multipart/byteranges
+# multipart/digest
+# multipart/encrypted
+# multipart/example
+# multipart/form-data
+# multipart/header-set
+# multipart/mixed
+# multipart/parallel
+# multipart/related
+# multipart/report
+# multipart/signed
+# multipart/voice-message
+# multipart/x-mixed-replace
+# text/1d-interleaved-parityfec
+text/cache-manifest appcache
+text/calendar ics ifb
+text/css css
+text/csv csv
+# text/csv-schema
+# text/directory
+# text/dns
+# text/ecmascript
+# text/encaprtp
+# text/enriched
+# text/example
+# text/fwdred
+# text/grammar-ref-list
+text/html html htm
+text/javascript js mjs
+# text/jcr-cnd
+# text/markdown
+# text/mizar
+text/n3 n3
+# text/parameters
+# text/parityfec
+text/plain txt text conf def list log in
+# text/provenance-notation
+# text/prs.fallenstein.rst
+text/prs.lines.tag dsc
+# text/prs.prop.logic
+# text/raptorfec
+# text/red
+# text/rfc822-headers
+text/richtext rtx
+# text/rtf
+# text/rtp-enc-aescm128
+# text/rtploopback
+# text/rtx
+text/sgml sgml sgm
+# text/t140
+text/tab-separated-values tsv
+text/troff t tr roff man me ms
+text/turtle ttl
+# text/ulpfec
+text/uri-list uri uris urls
+text/vcard vcard
+# text/vnd.a
+# text/vnd.abc
+text/vnd.curl curl
+text/vnd.curl.dcurl dcurl
+text/vnd.curl.mcurl mcurl
+text/vnd.curl.scurl scurl
+# text/vnd.debian.copyright
+# text/vnd.dmclientscript
+text/vnd.dvb.subtitle sub
+# text/vnd.esmertec.theme-descriptor
+text/vnd.fly fly
+text/vnd.fmi.flexstor flx
+text/vnd.graphviz gv
+text/vnd.in3d.3dml 3dml
+text/vnd.in3d.spot spot
+# text/vnd.iptc.newsml
+# text/vnd.iptc.nitf
+# text/vnd.latex-z
+# text/vnd.motorola.reflex
+# text/vnd.ms-mediapackage
+# text/vnd.net2phone.commcenter.command
+# text/vnd.radisys.msml-basic-layout
+# text/vnd.si.uricatalogue
+text/vnd.sun.j2me.app-descriptor jad
+# text/vnd.trolltech.linguist
+# text/vnd.wap.si
+# text/vnd.wap.sl
+text/vnd.wap.wml wml
+text/vnd.wap.wmlscript wmls
+text/x-asm s asm
+text/x-c c cc cxx cpp h hh dic
+text/x-fortran f for f77 f90
+text/x-java-source java
+text/x-nfo nfo
+text/x-opml opml
+text/x-pascal p pas
+text/x-setext etx
+text/x-sfv sfv
+text/x-uuencode uu
+text/x-vcalendar vcs
+text/x-vcard vcf
+# text/xml
+# text/xml-external-parsed-entity
+# video/1d-interleaved-parityfec
+video/3gpp 3gp
+# video/3gpp-tt
+video/3gpp2 3g2
+# video/bmpeg
+# video/bt656
+# video/celb
+# video/dv
+# video/encaprtp
+# video/example
+video/h261 h261
+video/h263 h263
+# video/h263-1998
+# video/h263-2000
+video/h264 h264
+# video/h264-rcdo
+# video/h264-svc
+# video/h265
+# video/iso.segment
+video/jpeg jpgv
+# video/jpeg2000
+video/jpm jpm jpgm
+video/mj2 mj2 mjp2
+# video/mp1s
+# video/mp2p
+# video/mp2t
+video/mp4 mp4 mp4v mpg4
+# video/mp4v-es
+video/mpeg mpeg mpg mpe m1v m2v
+# video/mpeg4-generic
+# video/mpv
+# video/nv
+video/ogg ogv
+# video/parityfec
+# video/pointer
+video/quicktime qt mov
+# video/raptorfec
+# video/raw
+# video/rtp-enc-aescm128
+# video/rtploopback
+# video/rtx
+# video/smpte292m
+# video/ulpfec
+# video/vc1
+# video/vnd.cctv
+video/vnd.dece.hd uvh uvvh
+video/vnd.dece.mobile uvm uvvm
+# video/vnd.dece.mp4
+video/vnd.dece.pd uvp uvvp
+video/vnd.dece.sd uvs uvvs
+video/vnd.dece.video uvv uvvv
+# video/vnd.directv.mpeg
+# video/vnd.directv.mpeg-tts
+# video/vnd.dlna.mpeg-tts
+video/vnd.dvb.file dvb
+video/vnd.fvt fvt
+# video/vnd.hns.video
+# video/vnd.iptvforum.1dparityfec-1010
+# video/vnd.iptvforum.1dparityfec-2005
+# video/vnd.iptvforum.2dparityfec-1010
+# video/vnd.iptvforum.2dparityfec-2005
+# video/vnd.iptvforum.ttsavc
+# video/vnd.iptvforum.ttsmpeg2
+# video/vnd.motorola.video
+# video/vnd.motorola.videop
+video/vnd.mpegurl mxu m4u
+video/vnd.ms-playready.media.pyv pyv
+# video/vnd.nokia.interleaved-multimedia
+# video/vnd.nokia.videovoip
+# video/vnd.objectvideo
+# video/vnd.radgamettools.bink
+# video/vnd.radgamettools.smacker
+# video/vnd.sealed.mpeg1
+# video/vnd.sealed.mpeg4
+# video/vnd.sealed.swf
+# video/vnd.sealedmedia.softseal.mov
+video/vnd.uvvu.mp4 uvu uvvu
+video/vnd.vivo viv
+# video/vp8
+video/webm webm
+video/x-f4v f4v
+video/x-fli fli
+video/x-flv flv
+video/x-m4v m4v
+video/x-matroska mkv mk3d mks
+video/x-mng mng
+video/x-ms-asf asf asx
+video/x-ms-vob vob
+video/x-ms-wm wm
+video/x-ms-wmv wmv
+video/x-ms-wmx wmx
+video/x-ms-wvx wvx
+video/x-msvideo avi
+video/x-sgi-movie movie
+video/x-smv smv
+x-conference/x-cooltalk ice
diff --git a/data-models/japanese-user-context.jsonld b/data-models/japanese-user-context.jsonld
new file mode 100644
index 0000000..804c0dc
--- /dev/null
+++ b/data-models/japanese-user-context.jsonld
@@ -0,0 +1,31 @@
+{
+ "@context": {
+ "タイプ": "@type",
+ "識別子": "@id",
+ "schema": "https://schema.org/",
+ "fiware": "https://uri.fiware.org/ns/data-models#",
+ "core": "https://uri.etsi.org/ngsi-ld/",
+ "tutorial": "https://fiware.github.io/tutorials.Step-by-Step/schema/",
+ "openstreetmap": "https://wiki.openstreetmap.org/wiki/Tag:building%3D",
+ "ビル": "fiware:Building",
+ "住所": "schema:address",
+ "コマーシャル": "openstreetmap:commercial",
+ "オフィス": "openstreetmap:office",
+ "インダストリアル": "openstreetmap:industrial",
+ "リテール": "openstreetmap:retail",
+ "レジデンシャル": "openstreetmap:residential",
+ "カテゴリー": "fiware:category",
+ "検証済み": "tutorial:verified",
+ "家具": "tutorial:furniture",
+ "名前": "schema:name",
+
+ "値": "core:hasValue",
+ "オブジェクト": "core:hasObject",
+
+ "座標": "core:coordinates",
+ "場所": "core:location",
+ "プロパティ": "core:Property",
+ "プロパティ": "core:Relationship",
+ "ジオプロパティ": "core:GeoProperty"
+ }
+}
diff --git a/data-models/ngsi-context.jsonld b/data-models/ngsi-context.jsonld
new file mode 100644
index 0000000..c9fbd3f
--- /dev/null
+++ b/data-models/ngsi-context.jsonld
@@ -0,0 +1,6 @@
+{
+ "@context": [
+ "http://context/user-context.jsonld",
+ "https://uri.etsi.org/ngsi-ld/v1/ngsi-ld-core-context-v1.7.jsonld"
+ ]
+}
diff --git a/data-models/user-context-with-graph.jsonld b/data-models/user-context-with-graph.jsonld
new file mode 100644
index 0000000..4fee875
--- /dev/null
+++ b/data-models/user-context-with-graph.jsonld
@@ -0,0 +1,493 @@
+{
+ "@context": {
+ "type": "@type",
+ "id": "@id",
+ "ngsi-ld": "https://uri.etsi.org/ngsi-ld/",
+ "fiware": "https://uri.fiware.org/ns/data-models#",
+ "schema": "https://schema.org/",
+ "tutorial": "https://fiware.github.io/tutorials.Step-by-Step/schema/",
+ "openstreetmap": "https://wiki.openstreetmap.org/wiki/Tag:building%3D",
+ "Bell": "tutorial:Bell",
+ "Building": "fiware:Building",
+ "Door": "tutorial:Door",
+ "Lamp": "tutorial:Lamp",
+ "Motion": "tutorial:Motion",
+ "Person": "fiware:Person",
+ "Product": "tutorial:Product",
+ "Shelf": "tutorial:Shelf",
+ "StockOrder": "tutorial:StockOrder",
+ "additionalName": "schema:additionalName",
+ "address": "schema:address",
+ "addressCountry": "schema:addressCountry",
+ "addressLocality": "schema:addressLocality",
+ "addressRegion": "schema:addressRegion",
+ "batteryLevel": "fiware:batteryLevel",
+ "category": "fiware:category",
+ "close": "tutorial:close",
+ "close_info": "tutorial:close_info",
+ "close_status": "tutorial:close_status",
+ "commercial": "openstreetmap:commercial",
+ "completed": "tutorial:completed",
+ "configuration": "fiware:configuration",
+ "containedInPlace": "fiware:containedInPlace",
+ "controlledAsset": "fiware:controlledAsset",
+ "controlledProperty": "fiware:controlledProperty",
+ "coordinates": "ngsi-ld:coordinates",
+ "currency": "tutorial:currency",
+ "dataProvider": "fiware:dataProvider",
+ "dateCreated": "fiware:dateCreated",
+ "dateFirstUsed": "fiware:dateFirstUsed",
+ "dateInstalled": "fiware:dateInstalled",
+ "dateLastCalibration": "fiware:dateLastCalibration",
+ "dateLastValueReported": "fiware:dateLastValueReported",
+ "dateManufactured": "fiware:dateManufactured",
+ "dateModified": "fiware:dateModified",
+ "description": "ngsi-ld:description",
+ "deviceState": "fiware:deviceState",
+ "email": "schema:email",
+ "familyName": "schema:familyName",
+ "faxNumber": "schema:faxNumber",
+ "firmwareVersion": "fiware:firmwareVersion",
+ "floorsAboveGround": "fiware:floorsAboveGround",
+ "floorsBelowGround": "fiware:floorsBelowGround",
+ "furniture": "tutorial:furniture",
+ "gender": "schema:gender",
+ "givenName": "schema:givenName",
+ "hardwareVersion": "fiware:hardwareVersion",
+ "honorificPrefix": "schema:honorificPrefix",
+ "honorificSuffix": "schema:honorificSuffix",
+ "humidity": "https://w3id.org/saref#humidity",
+ "inProgress": "tutorial:inProgress",
+ "industrial": "openstreetmap:industrial",
+ "installedBy": "tutorial:installedBy",
+ "ipAddress": "fiware:ipAddress",
+ "isicV4": "schema:isicV4",
+ "jobTitle": "schema:jobTitle",
+ "kiosk": "openstreetmap:kiosk",
+ "locatedIn": "tutorial:locatedIn",
+ "location": "https://w3id.org/saref#location",
+ "lock": "tutorial:lock",
+ "lock_info": "tutorial:lock_info",
+ "lock_status": "tutorial:lock_status",
+ "luminiscence": "https://w3id.org/saref#luminiscence",
+ "macAddress": "fiware:macAddress",
+ "mcc": "fiware:mcc",
+ "mnc": "fiware:mnc",
+ "motion": "https://w3id.org/saref#motion",
+ "name": "schema:name",
+ "numberOfItems": "tutorial:numberOfItems",
+ "observedAt": "ngsi-ld:observedAt",
+ "occupier": "fiware:occupier",
+ "off": "tutorial:off",
+ "off_info": "tutorial:off_info",
+ "off_status": "tutorial:off_status",
+ "office": "openstreetmap:office",
+ "off": "tutorial:off",
+ "on_info": "tutorial:on_info",
+ "on_status": "tutorial:on_status",
+ "open": "tutorial:open",
+ "open_info": "tutorial:open_info",
+ "open_status": "tutorial:open_status",
+ "openingHours": "fiware:openingHours",
+ "orderDate": "tutorial:orderDate",
+ "orderedProduct": "tutorial:orderedProduct",
+ "osVersion": "fiware:osVersion",
+ "owner": "fiware:owner",
+ "pending": "tutorial:pending",
+ "postOfficeBoxNumber": "schema:postOfficeBoxNumber",
+ "postalCode": "schema:postalCode",
+ "price": "tutorial:price",
+ "providedBy": "fiware:providedBy",
+ "provider": "fiware:provider",
+ "refDeviceModel": "fiware:refDeviceModel",
+ "refMap": "fiware:refMap",
+ "requestedBy": "tutorial:requestedBy",
+ "requestedFor": "tutorial:requestedFor",
+ "retail": "openstreetmap:retail",
+ "ring": "tutorial:ring",
+ "ring_info": "tutorial:ring_info",
+ "ring_status": "tutorial:ring_status",
+ "rssi": "fiware:rssi",
+ "serialNumber": "fiware:serialNumber",
+ "size": "tutorial:size",
+ "softwareVersion": "fiware:softwareVersion",
+ "source": "fiware:source",
+ "statusOfWork": "tutorial:statusOfWork",
+ "stockCount": "tutorial:stockCount",
+ "stocks": "tutorial:stocks",
+ "streetAddress": "schema:streetAddress",
+ "supermarket": "openstreetmap:supermarket",
+ "supportedProtocol": "fiware:supportedProtocol",
+ "taxID": "schema:taxID",
+ "telephone": "schema:telephone",
+ "temperature": "https://w3id.org/saref#temperature",
+ "tweets": "tutorial:tweets",
+ "unitCode": "ngsi-ld:unitCode",
+ "unlock": "tutorial:unlock",
+ "unlock_info": "tutorial:unlock_info",
+ "unlock_status": "tutorial:unlock_status",
+ "value": "fiware:value",
+ "vatID": "schema:vatID",
+ "verified": "tutorial:verified",
+ "warehouse": "openstreetmap:warehouse"
+ },
+ "@graph": [
+ {
+ "@id": "tutorial:Product",
+ "@type": "rdfs:Class",
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "Product is something sold in a Store."
+ },
+ {
+ "@language": "ja",
+ "@value": "製品はストアで販売されている物"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "Product"
+ },
+ {
+ "@language": "ja",
+ "@value": "製品"
+ }
+ ],
+ "rdfs:subClassOf": {
+ "@id": "http://schema.org/Thing"
+ }
+ },
+ {
+ "@id": "tutorial:Shelf",
+ "@type": "rdfs:Class",
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "A Shelf is a unit of Store Furniture"
+ },
+ {
+ "@language": "ja",
+ "@value": "棚はストア用家具のユニット"
+ }
+ ],
+ "rdfs:label":
+ [
+ {
+ "@language": "en",
+ "@value": "Shelf"
+ },
+ {
+ "@language": "ja",
+ "@value": "棚"
+ }
+ ],
+ "rdfs:subClassOf": {
+ "@id": "http://schema.org/Thing"
+ }
+ },
+ {
+ "@id": "tutorial:StockOrder",
+ "@type": "rdfs:Class",
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "StockOrder an entry in a list of items sold in one or more stores"
+ },
+ {
+ "@language": "ja",
+ "@value": "在庫注文は1つ以上のストアで販売されるアイテムの一覧のエントリ"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "StockOrder"
+ },
+ {
+ "@language": "ja",
+ "@value": "在庫注文"
+ }
+ ],
+ "rdfs:subClassOf": {
+ "@id": "http://schema.org/Intangible"
+ }
+ },
+ {
+ "@id": "schema:Person",
+ "@type": "rdfs:Class",
+ "http://purl.org/dc/terms/source": {
+ "@id": "http://www.w3.org/wiki/WebSchemas/SchemaDotOrgSources#source_rNews"
+ },
+ "http://www.w3.org/2002/07/owl#equivalentClass": {
+ "@id": "http://xmlns.com/foaf/0.1/Person"
+ },
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "A person (alive, dead, undead, or fictional)."
+ },
+ {
+ "@language": "ja",
+ "@value": "人 (生きている, 死んでいる, 完全に死んでいない, または架空の人)"
+ }
+ ],
+ "rdfs:label":
+ [
+ {
+ "@language": "en",
+ "@value": "Person"
+ },
+ {
+ "@language": "ja",
+ "@value": "人"
+ }
+ ],
+ "rdfs:subClassOf": {
+ "@id": "http://schema.org/Thing"
+ }
+ },
+ {
+ "@id": "tutoral:requestedBy",
+ "@type": "https://uri.etsi.org/ngsi-ld/Relationship",
+ "schema:domainIncludes": [
+ {
+ "@id": "tutorial:Shelf"
+ },
+ {
+ "@id": "tutorial:StockOrder"
+ }
+ ],
+ "schema:rangeIncludes": [
+ {
+ "@id": "schema:Person"
+ }
+ ],
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "Object requested by person."
+ },
+ {
+ "@language": "ja",
+ "@value": "人が要求したオブジェクト"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "requestedBy"
+ },
+ {
+ "@language": "ja",
+ "@value": "要求者"
+ }
+ ]
+ },
+ {
+ "@id": "tutoral:installedBy",
+ "@type": "https://uri.etsi.org/ngsi-ld/Relationship",
+ "schema:domainIncludes": [
+ {
+ "@id": "tutorial:Shelf"
+ }
+ ],
+ "schema:rangeIncludes": [
+ {
+ "@id": "schema:Person"
+ }
+ ],
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "Person who installs an Object"
+ },
+ {
+ "@language": "ja",
+ "@value": "オブジェクトを設置する人"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "installedBy"
+ },
+ {
+ "@language": "ja",
+ "@value": "設置者"
+ }
+ ]
+ },
+ {
+ "@id": "tutorial:furniture",
+ "@type": "https://uri.etsi.org/ngsi-ld/Relationship",
+ "schema:domainIncludes": [
+ {
+ "@id": "fiware:Building"
+ }
+ ],
+ "schema:rangeIncludes": [
+ {
+ "@id": "tutorial:Shelf"
+ }
+ ],
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "Units found within a Building"
+ },
+ {
+ "@language": "ja",
+ "@value": "建物内で見つかったユニット"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "furniture"
+ },
+ {
+ "@language": "ja",
+ "@value": "家具"
+ }
+ ]
+ },
+ {
+ "@id": "tutorial:locatedIn",
+ "@type": "https://uri.etsi.org/ngsi-ld/Relationship",
+ "schema:domainIncludes": [
+ {
+ "@id": "tutorial:Shelf"
+ }
+ ],
+ "schema:rangeIncludes": [
+ {
+ "@id": "fiware:Building"
+ }
+ ],
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "Building in which an item is found"
+ },
+ {
+ "@language": "ja",
+ "@value": "アイテムが見つかった建物"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "locatedIn"
+ },
+ {
+ "@language": "ja",
+ "@value": "置き場所"
+ }
+ ]
+ },
+ {
+ "@id": "tutorial:stocks",
+ "@type": "https://uri.etsi.org/ngsi-ld/Relationship",
+ "schema:domainIncludes": [
+ {
+ "@id": "tutorial:Shelf"
+ }
+ ],
+ "schema:rangeIncludes": [
+ {
+ "@id": "tutorial:Product"
+ }
+ ],
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "The product found on a shelf"
+ },
+ {
+ "@language": "ja",
+ "@value": "棚の上にある製品"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "stocks"
+ },
+ {
+ "@language": "ja",
+ "@value": "在庫"
+ }
+ ]
+ },
+ {
+ "@id": "tutorial:requestedFor",
+ "@type": "https://uri.etsi.org/ngsi-ld/Relationship",
+ "schema:domainIncludes": [
+ {
+ "@id": "tutorial:StockOrder"
+ }
+ ],
+ "schema:rangeIncludes": [
+ {
+ "@id": "fiware:Building"
+ }
+ ],
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "Store for which an item is requested"
+ },
+ {
+ "@language": "ja",
+ "@value": "アイテムが要求されたストア"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "requestedFor"
+ },
+ {
+ "@language": "ja",
+ "@value": "要求対象"
+ }
+ ]
+ },
+ {
+ "@id": "tutorial:orderedProduct",
+ "@type": "https://uri.etsi.org/ngsi-ld/Relationship",
+ "schema:domainIncludes": [
+ {
+ "@id": "tutorial:StockOrder"
+ }
+ ],
+ "schema:rangeIncludes": [
+ {
+ "@id": "tutorial:Product"
+ }
+ ],
+ "rdfs:comment": [
+ {
+ "@language": "en",
+ "@value": "The Product ordered for a store"
+ },
+ {
+ "@language": "ja",
+ "@value": "ストアで注文された製品"
+ }
+ ],
+ "rdfs:label": [
+ {
+ "@language": "en",
+ "@value": "orderedProduct"
+ },
+ {
+ "@language": "ja",
+ "@value": "注文された製品"
+ }
+ ]
+ }
+ ]
+}
diff --git a/data-models/user-context.jsonld b/data-models/user-context.jsonld
new file mode 100644
index 0000000..ab6f9b1
--- /dev/null
+++ b/data-models/user-context.jsonld
@@ -0,0 +1,134 @@
+{
+ "@context": {
+ "type": "@type",
+ "id": "@id",
+ "ngsi-ld": "https://uri.etsi.org/ngsi-ld/",
+ "fiware": "https://uri.fiware.org/ns/data-models#",
+ "schema": "https://schema.org/",
+ "tutorial": "https://fiware.github.io/tutorials.Step-by-Step/schema/",
+ "openstreetmap": "https://wiki.openstreetmap.org/wiki/Tag:building%3D",
+ "Bell": "tutorial:Bell",
+ "Building": "fiware:Building",
+ "Door": "tutorial:Door",
+ "Lamp": "tutorial:Lamp",
+ "Motion": "tutorial:Motion",
+ "Person": "fiware:Person",
+ "Product": "tutorial:Product",
+ "Shelf": "tutorial:Shelf",
+ "StockOrder": "tutorial:StockOrder",
+ "additionalName": "schema:additionalName",
+ "address": "schema:address",
+ "addressCountry": "schema:addressCountry",
+ "addressLocality": "schema:addressLocality",
+ "addressRegion": "schema:addressRegion",
+ "batteryLevel": "fiware:batteryLevel",
+ "category": "fiware:category",
+ "close": "tutorial:close",
+ "close_info": "tutorial:close_info",
+ "close_status": "tutorial:close_status",
+ "commercial": "openstreetmap:commercial",
+ "completed": "tutorial:completed",
+ "configuration": "fiware:configuration",
+ "containedInPlace": "fiware:containedInPlace",
+ "controlledAsset": "fiware:controlledAsset",
+ "controlledProperty": "fiware:controlledProperty",
+ "coordinates": "ngsi-ld:coordinates",
+ "currency": "tutorial:currency",
+ "dataProvider": "fiware:dataProvider",
+ "dateCreated": "fiware:dateCreated",
+ "dateFirstUsed": "fiware:dateFirstUsed",
+ "dateInstalled": "fiware:dateInstalled",
+ "dateLastCalibration": "fiware:dateLastCalibration",
+ "dateLastValueReported": "fiware:dateLastValueReported",
+ "dateManufactured": "fiware:dateManufactured",
+ "dateModified": "fiware:dateModified",
+ "description": "ngsi-ld:description",
+ "deviceState": "fiware:deviceState",
+ "email": "schema:email",
+ "familyName": "schema:familyName",
+ "faxNumber": "schema:faxNumber",
+ "firmwareVersion": "fiware:firmwareVersion",
+ "floorsAboveGround": "fiware:floorsAboveGround",
+ "floorsBelowGround": "fiware:floorsBelowGround",
+ "furniture": "tutorial:furniture",
+ "gender": "schema:gender",
+ "givenName": "schema:givenName",
+ "hardwareVersion": "fiware:hardwareVersion",
+ "honorificPrefix": "schema:honorificPrefix",
+ "honorificSuffix": "schema:honorificSuffix",
+ "humidity": "https://w3id.org/saref#humidity",
+ "inProgress": "tutorial:inProgress",
+ "industrial": "openstreetmap:industrial",
+ "installedBy": "tutorial:installedBy",
+ "ipAddress": "fiware:ipAddress",
+ "isicV4": "schema:isicV4",
+ "jobTitle": "schema:jobTitle",
+ "kiosk": "openstreetmap:kiosk",
+ "locatedIn": "tutorial:locatedIn",
+ "location": "https://w3id.org/saref#location",
+ "lock": "tutorial:lock",
+ "lock_info": "tutorial:lock_info",
+ "lock_status": "tutorial:lock_status",
+ "luminiscence": "https://w3id.org/saref#luminiscence",
+ "macAddress": "fiware:macAddress",
+ "mcc": "fiware:mcc",
+ "mnc": "fiware:mnc",
+ "motion": "https://w3id.org/saref#motion",
+ "name": "schema:name",
+ "numberOfItems": "tutorial:numberOfItems",
+ "observedAt": "ngsi-ld:observedAt",
+ "occupier": "fiware:occupier",
+ "off": "tutorial:off",
+ "off_info": "tutorial:off_info",
+ "off_status": "tutorial:off_status",
+ "office": "openstreetmap:office",
+ "off": "tutorial:off",
+ "on_info": "tutorial:on_info",
+ "on_status": "tutorial:on_status",
+ "open": "tutorial:open",
+ "open_info": "tutorial:open_info",
+ "open_status": "tutorial:open_status",
+ "openingHours": "fiware:openingHours",
+ "orderDate": "tutorial:orderDate",
+ "orderedProduct": "tutorial:orderedProduct",
+ "osVersion": "fiware:osVersion",
+ "owner": "fiware:owner",
+ "pending": "tutorial:pending",
+ "postOfficeBoxNumber": "schema:postOfficeBoxNumber",
+ "postalCode": "schema:postalCode",
+ "price": "tutorial:price",
+ "providedBy": "fiware:providedBy",
+ "provider": "fiware:provider",
+ "refDeviceModel": "fiware:refDeviceModel",
+ "refMap": "fiware:refMap",
+ "requestedBy": "tutorial:requestedBy",
+ "requestedFor": "tutorial:requestedFor",
+ "retail": "openstreetmap:retail",
+ "ring": "tutorial:ring",
+ "ring_info": "tutorial:ring_info",
+ "ring_status": "tutorial:ring_status",
+ "rssi": "fiware:rssi",
+ "serialNumber": "fiware:serialNumber",
+ "size": "tutorial:size",
+ "softwareVersion": "fiware:softwareVersion",
+ "source": "fiware:source",
+ "statusOfWork": "tutorial:statusOfWork",
+ "stockCount": "tutorial:stockCount",
+ "stocks": "tutorial:stocks",
+ "streetAddress": "schema:streetAddress",
+ "supermarket": "openstreetmap:supermarket",
+ "supportedProtocol": "fiware:supportedProtocol",
+ "taxID": "schema:taxID",
+ "telephone": "schema:telephone",
+ "temperature": "https://w3id.org/saref#temperature",
+ "tweets": "tutorial:tweets",
+ "unitCode": "ngsi-ld:unitCode",
+ "unlock": "tutorial:unlock",
+ "unlock_info": "tutorial:unlock_info",
+ "unlock_status": "tutorial:unlock_status",
+ "value": "fiware:value",
+ "vatID": "schema:vatID",
+ "verified": "tutorial:verified",
+ "warehouse": "openstreetmap:warehouse"
+ }
+}
diff --git a/docker-compose/common.yml b/docker-compose/common.yml
index 5eceea5..2342d81 100644
--- a/docker-compose/common.yml
+++ b/docker-compose/common.yml
@@ -17,7 +17,25 @@
# see: https://github.com/FIWARE/helm-charts/
#
version: "3.8"
-services:
+services:
+ # @context file is served from here
+ ld-context:
+ labels:
+ org.fiware: 'tutorial'
+ image: httpd:alpine
+ hostname: context
+ container_name: fiware-ld-context
+ ports:
+ - "3004:80"
+ volumes:
+ - data-models:/usr/local/apache2/htdocs/
+ - type: bind
+ source: ${PWD}/conf/mime.types
+ target: /usr/local/apache2/conf/mime.types
+ read_only: true
+ healthcheck:
+ test: (wget --server-response --spider --quiet http://context/ngsi-context.jsonld 2>&1 | awk 'NR==1{print $$2}'| grep -q -e "200") || exit 1
+
# Databases
mongo-db:
labels:
@@ -66,19 +84,6 @@ services:
- "WEB_APP_PORT=${TUTORIAL_APP_PORT}" # Port used by the content provider proxy and web-app for viewing data
- "NGSI_VERSION=ngsi-ld"
- "CONTEXT_BROKER=http://orion:${ORION_LD_PORT}/ngsi-ld/v1" # URL of the context broker to update context
- - "DEVICE_BROKER=http://devices:${ORION_EDGE_PORT}/v2" # URL of the device's context broker to update context
- - "NGSI_LD_PREFIX=urn:ngsi-ld:"
-
- - "IOTA_HTTP_HOST=iot-agent"
- - "IOTA_HTTP_PORT=${IOTA_SOUTH_PORT}"
- - "DUMMY_DEVICES_PORT=${TUTORIAL_DUMMY_DEVICE_PORT}" # Port used by the dummy IOT devices to receive commands
- - "DUMMY_DEVICES_TRANSPORT=HTTP" # Default transport used by dummy Io devices
-
- - "OPENWEATHERMAP_KEY_ID="
- - "TWITTER_CONSUMER_KEY="
- - "TWITTER_CONSUMER_SECRET="
-
-
networks:
default:
@@ -87,3 +92,9 @@ networks:
volumes:
mongo-db: ~
+ data-models:
+ driver: local
+ driver_opts:
+ type: none
+ o: bind
+ device: ${PWD}/data-models
diff --git a/docker-compose/orion-ld.yml b/docker-compose/orion-ld.yml
index 719bb11..0f0de25 100644
--- a/docker-compose/orion-ld.yml
+++ b/docker-compose/orion-ld.yml
@@ -30,8 +30,8 @@ services:
networks:
- default
ports:
- - "${ORION_LD_PORT}:${ORION_LD_PORT}" # localhost:1026
- command: -dbhost mongo-db -logLevel DEBUG -forwarding
+ - "${EXPOSED_PORT}:${ORION_LD_PORT}" # localhost:1026
+ command: -dbhost mongo-db -logLevel DEBUG -experimental
healthcheck:
test: curl --fail -s http://orion:${ORION_LD_PORT}/version || exit 1
interval: 5s
diff --git a/docker-compose/stellio.yml b/docker-compose/stellio.yml
index 095d17a..b834e97 100644
--- a/docker-compose/stellio.yml
+++ b/docker-compose/stellio.yml
@@ -27,7 +27,7 @@ services:
environment:
- SPRING_PROFILES_ACTIVE=docker
ports:
- - "${STELLIO_PORT}:8080"
+ - "${EXPOSED_PORT}:${STELLIO_PORT}"
networks:
- default
diff --git a/import-data b/import-data
index d9059dd..60c6793 100755
--- a/import-data
+++ b/import-data
@@ -20,7 +20,7 @@ curl -s -o /dev/null -X POST \
-d '{
"id": "urn:ngsi-ld:Building:store001",
"type": "Building",
- "category": {"type": "Property", "value": ["commercial"]},
+ "category": {"type": "VocabularyProperty", "vocab": "commercial"},
"address": {
"type": "Property",
"value": {"streetAddress": "Bornholmer Straße 65", "addressRegion": "Berlin", "addressLocality": "Prenzlauer Berg", "postalCode": "10439"},
@@ -49,7 +49,7 @@ curl -s -o /dev/null -X POST \
-d '{
"id": "urn:ngsi-ld:Building:store002",
"type": "Building",
- "category": {"type": "Property", "value": ["commercial"]},
+ "category": {"type": "VocabularyProperty", "vocab": ["commercial"]},
"address": {
"type": "Property",
"value": {"streetAddress":"Friedrichstraße 44", "addressRegion":"Berlin", "addressLocality":"Kreuzberg", "postalCode":"10969"},
@@ -74,7 +74,7 @@ curl -s -o /dev/null -X POST \
-d '{
"id": "urn:ngsi-ld:Building:store003",
"type": "Building",
- "category": {"type": "Property", "value": ["commercial"]},
+ "category": {"type": "VocabularyProperty", "vocab": ["commercial"]},
"address": {
"type": "Property",
"value": {"streetAddress":"Mühlenstrasse 10", "addressRegion":"Berlin", "addressLocality":"Friedrichshain", "postalCode":"10243"},
@@ -101,7 +101,7 @@ curl -s -o /dev/null -X POST \
-d '{
"id": "urn:ngsi-ld:Building:store004",
"type": "Building",
- "category": {"type": "Property", "value": ["commercial"]},
+ "category": {"type": "VocabularyProperty", "vocab": ["commercial"]},
"address": {
"type": "Property",
"value": {"streetAddress":"Panoramastraße 1A", "addressRegion":"Berlin", "addressLocality":"Mitte", "postalCode":"10178"},
diff --git a/services b/services
index faaf1af..cfcfc6e 100755
--- a/services
+++ b/services
@@ -10,8 +10,8 @@ set -e
SCORPIO="http://scorpio:9090/"
ORION="http://orion:1026/version"
STELLIO="http://localhost:8080/actuator/health"
-CONTEXT="https://fiware.github.io/tutorials.Step-by-Step/tutorials-context.jsonld"
-CORE_CONTEXT="https://uri.etsi.org/ngsi-ld/v1/ngsi-ld-core-context.jsonld"
+CONTEXT="http://context/ngsi-context.jsonld"
+CORE_CONTEXT="https://uri.etsi.org/ngsi-ld/v1/ngsi-ld-core-context-v1.7.jsonld"
dockerCmd="docker compose"
if (( $# == 2 )); then